springboot多数据源配置代码实现
时间: 2023-11-08 10:58:43 浏览: 121
在Spring Boot中配置多个数据源可以使用以下步骤:
1. 配置数据源
```java
@Configuration
public class DataSourceConfig {
@Bean
@ConfigurationProperties(prefix = "spring.datasource.primary")
public DataSource primaryDataSource() {
return DataSourceBuilder.create().build();
}
@Bean
@ConfigurationProperties(prefix = "spring.datasource.secondary")
public DataSource secondaryDataSource() {
return DataSourceBuilder.create().build();
}
}
```
2. 配置事务管理器
```java
@Configuration
@EnableTransactionManagement
public class TransactionConfig {
@Autowired
private DataSource primaryDataSource;
@Autowired
private DataSource secondaryDataSource;
@Bean(name = "primaryTransactionManager")
public PlatformTransactionManager primaryTransactionManager() {
return new DataSourceTransactionManager(primaryDataSource);
}
@Bean(name = "secondaryTransactionManager")
public PlatformTransactionManager secondaryTransactionManager() {
return new DataSourceTransactionManager(secondaryDataSource);
}
@Bean(name = "primaryJdbcTemplate")
public JdbcTemplate primaryJdbcTemplate(@Qualifier("primaryDataSource") DataSource dataSource) {
return new JdbcTemplate(dataSource);
}
@Bean(name = "secondaryJdbcTemplate")
public JdbcTemplate secondaryJdbcTemplate(@Qualifier("secondaryDataSource") DataSource dataSource) {
return new JdbcTemplate(dataSource);
}
}
```
3. 配置JPA
```java
@Configuration
@EnableJpaRepositories(
entityManagerFactoryRef = "primaryEntityManagerFactory",
transactionManagerRef = "primaryTransactionManager",
basePackages = {"com.example.primary.repository"})
public class PrimaryDataSourceConfig {
@Autowired
private Environment env;
@Primary
@Bean(name = "primaryDataSource")
public DataSource primaryDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName(env.getProperty("spring.datasource.primary.driverClassName"));
dataSource.setUrl(env.getProperty("spring.datasource.primary.url"));
dataSource.setUsername(env.getProperty("spring.datasource.primary.username"));
dataSource.setPassword(env.getProperty("spring.datasource.primary.password"));
return dataSource;
}
@Primary
@Bean(name = "primaryEntityManagerFactory")
public LocalContainerEntityManagerFactoryBean primaryEntityManagerFactory(EntityManagerFactoryBuilder builder,
@Qualifier("primaryDataSource") DataSource dataSource) {
return builder
.dataSource(dataSource)
.packages("com.example.primary.entity")
.persistenceUnit("primary")
.build();
}
@Primary
@Bean(name = "primaryTransactionManager")
public PlatformTransactionManager primaryTransactionManager(
@Qualifier("primaryEntityManagerFactory") EntityManagerFactory primaryEntityManagerFactory) {
return new JpaTransactionManager(primaryEntityManagerFactory);
}
}
@Configuration
@EnableJpaRepositories(
entityManagerFactoryRef = "secondaryEntityManagerFactory",
transactionManagerRef = "secondaryTransactionManager",
basePackages = {"com.example.secondary.repository"})
public class SecondaryDataSourceConfig {
@Autowired
private Environment env;
@Bean(name = "secondaryDataSource")
public DataSource secondaryDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
dataSource.setDriverClassName(env.getProperty("spring.datasource.secondary.driverClassName"));
dataSource.setUrl(env.getProperty("spring.datasource.secondary.url"));
dataSource.setUsername(env.getProperty("spring.datasource.secondary.username"));
dataSource.setPassword(env.getProperty("spring.datasource.secondary.password"));
return dataSource;
}
@Bean(name = "secondaryEntityManagerFactory")
public LocalContainerEntityManagerFactoryBean secondaryEntityManagerFactory(EntityManagerFactoryBuilder builder,
@Qualifier("secondaryDataSource") DataSource dataSource) {
return builder
.dataSource(dataSource)
.packages("com.example.secondary.entity")
.persistenceUnit("secondary")
.build();
}
@Bean(name = "secondaryTransactionManager")
public PlatformTransactionManager secondaryTransactionManager(
@Qualifier("secondaryEntityManagerFactory") EntityManagerFactory secondaryEntityManagerFactory) {
return new JpaTransactionManager(secondaryEntityManagerFactory);
}
}
```
4. 配置application.properties
```properties
spring.datasource.primary.url=jdbc:mysql://localhost:3306/primary_db
spring.datasource.primary.username=root
spring.datasource.primary.password=root
spring.datasource.primary.driverClassName=com.mysql.jdbc.Driver
spring.datasource.secondary.url=jdbc:mysql://localhost:3306/secondary_db
spring.datasource.secondary.username=root
spring.datasource.secondary.password=root
spring.datasource.secondary.driverClassName=com.mysql.jdbc.Driver
```
完成以上步骤后,就可以在代码中使用多个数据源了。例如:
```java
@Service
public class UserService {
@Autowired
@Qualifier("primaryJdbcTemplate")
private JdbcTemplate primaryJdbcTemplate;
@Autowired
@Qualifier("secondaryJdbcTemplate")
private JdbcTemplate secondaryJdbcTemplate;
public void addUser(User user) {
String sql = "INSERT INTO user (username, password) VALUES (?, ?)";
primaryJdbcTemplate.update(sql, user.getUsername(), user.getPassword());
}
public List<User> getAllUsers() {
String sql = "SELECT * FROM user";
List<User> users = secondaryJdbcTemplate.query(sql, new BeanPropertyRowMapper<>(User.class));
return users;
}
}
```
以上就是使用Spring Boot实现多数据源配置的代码实现。
阅读全文
相关推荐
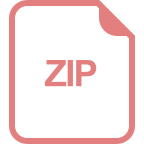
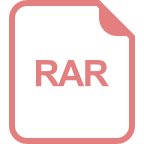
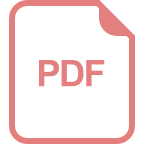














