用java写一个对Excel文件解析的代码,把每次读出的数据按照cell不同的位置存入到Map对象中
时间: 2023-05-21 22:01:16 浏览: 138
当然可以,以下是一个简单的Java代码示例,用于解析Excel文件并将数据存储在Map对象中:
```java
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelParser {
public static void main(String[] args) {
String filePath = "path/to/excel/file.xlsx";
Map<String, String> dataMap = parseExcel(filePath);
System.out.println(dataMap);
}
public static Map<String, String> parseExcel(String filePath) {
Map<String, String> dataMap = new HashMap<>();
try (FileInputStream inputStream = new FileInputStream(new File(filePath));
Workbook workbook = WorkbookFactory.create(inputStream)) {
Sheet sheet = workbook.getSheetAt(0);
for (Row row : sheet) {
Cell keyCell = row.getCell(0);
Cell valueCell = row.getCell(1);
if (keyCell != null && valueCell != null) {
String key = keyCell.getStringCellValue();
String value = valueCell.getStringCellValue();
dataMap.put(key, value);
}
}
} catch (IOException e) {
e.printStackTrace();
}
return dataMap;
}
}
```
这个代码示例使用了Apache POI库来解析Excel文件,将每个单元格的数据存储在一个Map对象中,其中Map的键是单元格的行号,值是单元格的值。你可以根据自己的需求修改代码来适应不同的Excel文件格式。
相关推荐
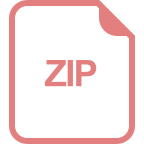
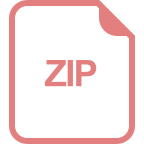
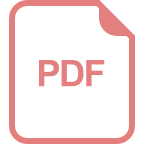














