对结构体中元素进行排序
时间: 2023-05-21 22:01:53 浏览: 84
可以使用 STL 中的 sort 函数对结构体中的元素进行排序。例如,假设有一个结构体 Person 包含姓名和年龄两个元素,可以按照年龄从小到大排序,代码如下:
```
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
struct Person {
string name;
int age;
};
bool cmp(Person a, Person b) {
return a.age < b.age;
}
int main() {
vector<Person> people = {{"Tom", 20}, {"Jerry", 18}, {"Alice", 22}};
sort(people.begin(), people.end(), cmp);
for (auto p : people) {
cout << p.name << " " << p.age << endl;
}
return 0;
}
```
输出结果为:
```
Jerry 18
Tom 20
Alice 22
```
其中,cmp 函数为自定义的比较函数,用于指定排序规则。在本例中,按照年龄从小到大排序。
相关问题
对结构体中元素从小到大进行排序
可以使用 STL 中的 sort 函数来对结构体中的元素从小到大进行排序。具体实现方法如下:
1. 定义结构体:
```
struct Student {
string name;
int age;
double score;
};
```
2. 定义比较函数:
```
bool cmp(const Student& a, const Student& b) {
return a.score < b.score;
}
```
3. 使用 sort 函数进行排序:
```
vector<Student> students = {{"Tom", 18, 90.5}, {"Jerry", 19, 85.5}, {"Alice", 20, 95.0}};
sort(students.begin(), students.end(), cmp);
```
这样就可以将结构体中的元素按照 score 从小到大进行排序了。
对结构体类型数据进行排序
可以使用标准库的`qsort`函数来对结构体类型数据进行排序。`qsort`函数的原型如下:
```c
void qsort(void *base, size_t nmemb, size_t size,
int (*compar)(const void *, const void *));
```
其中,`base`是待排序的数组首地址,`nmemb`是数组元素个数,`size`是数组每个元素的大小,`compar`是用来比较两个元素的函数指针。
假设有一个结构体类型为`Student`,其中包含`name`和`score`两个成员变量,可以通过如下的方式来定义比较函数:
```c
int compare(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
return s1->score - s2->score;
}
```
然后就可以使用`qsort`函数对`Student`类型的数组按照`score`成员变量进行排序了:
```c
Student students[] = {
{"Tom", 80},
{"Jerry", 70},
{"Alice", 90},
{"Bob", 85}
};
int n = sizeof(students) / sizeof(students[0]);
qsort(students, n, sizeof(Student), compare);
```
这样就可以将`students`数组按照`score`从小到大排序。
相关推荐
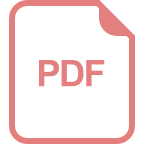
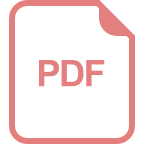












