c语言用qsort函数对结构体排序
时间: 2023-11-30 10:03:38 浏览: 42
假设有如下结构体:
```c
struct student{
char name[20];
int age;
float score;
};
```
可以通过以下代码使用 `qsort` 函数对结构体数组 `students` 按照分数从高到低进行排序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct student{
char name[20];
int age;
float score;
};
int cmp(const void *a, const void *b);
int main()
{
struct student students[5] = {{"Tom", 20, 86.5}, {"Jerry", 19, 92.0}, {"Alice", 21, 78.5}, {"Bob", 18, 95.0}, {"Ted", 20, 88.5}};
int i;
printf("Before sorting:\n");
for(i = 0; i < 5; i++){
printf("%s, %d, %.1f\n", students[i].name, students[i].age, students[i].score);
}
qsort(students, 5, sizeof(struct student), cmp);
printf("\nAfter sorting:\n");
for(i = 0; i < 5; i++){
printf("%s, %d, %.1f\n", students[i].name, students[i].age, students[i].score);
}
return 0;
}
int cmp(const void *a, const void *b)
{
struct student *s1 = (struct student *)a;
struct student *s2 = (struct student *)b;
if(s1->score < s2->score){
return 1;
}
else if(s1->score > s2->score){
return -1;
}
else{
return 0;
}
}
```
其中,`qsort` 函数的参数依次为:
- 待排序的数组名 `students`
- 数组中元素的个数 `5`
- 每个元素的大小 `sizeof(struct student)`
- 比较函数 `cmp`
`cmp` 函数用于定义两个元素之间的大小关系,返回值为负数表示第一个元素比第二个元素小,返回值为正数表示第一个元素比第二个元素大,返回值为零表示两个元素相等。在本例中,`cmp` 函数按照分数从高到低进行比较。
相关推荐
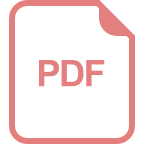
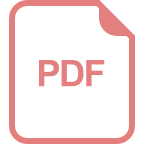
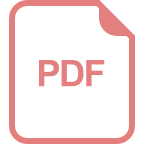














