怎么用qsort函数排序一个结构体,结构体包含学号,成绩,先按成绩排序,再按学号排序,用C语言写
时间: 2023-05-14 07:06:41 浏览: 117
可以按照以下步骤使用qsort函数对结构体进行排序:
1. 定义结构体类型,包含学号和成绩两个成员变量。
```
typedef struct {
int id; // 学号
int score; // 成绩
} Student;
```
2. 定义比较函数cmp,用于比较两个结构体的大小。
```
int cmp(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
if (s1->score != s2->score) {
return s2->score - s1->score; // 先按成绩从大到小排序
} else {
return s1->id - s2->id; // 如果成绩相同,则按学号从小到大排序
}
}
```
3. 定义结构体数组,初始化数据。
```
Student students[] = {
{1001, 90},
{1002, 80},
{1003, 85},
{1004, 95},
{1005, 85},
};
int n = sizeof(students) / sizeof(Student);
```
4. 调用qsort函数进行排序。
```
qsort(students, n, sizeof(Student), cmp);
```
5. 输出排序结果。
```
for (int i = 0; i < n; i++) {
printf("学号:%d,成绩:%d\n", students[i].id, students[i].score);
}
```
完整代码如下:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int id; // 学号
int score; // 成绩
} Student;
int cmp(const void *a, const void *b) {
Student *s1 = (Student *)a;
Student *s2 = (Student *)b;
if (s1->score != s2->score) {
return s2->score - s1->score; // 先按成绩从大到小排序
} else {
return s1->id - s2->id; // 如果成绩相同,则按学号从小到大排序
}
}
int main() {
Student students[] = {
{1001, 90},
{1002, 80},
{1003, 85},
{1004, 95},
{1005, 85},
};
int n = sizeof(students) / sizeof(Student);
qsort(students, n, sizeof(Student), cmp);
for (int i = 0; i < n; i++) {
printf("学号:%d,成绩:%d\n", students[i].id, students[i].score);
}
return 0;
}
```
阅读全文
相关推荐
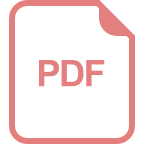















