实现公司员工的管理,设计employee类,包含员工基本信息:编号,姓名,性命,出生日期和职务等。出生日期使用自定义的date类,employee类有可能从外部访问类成员的友元函数,程序基本功能有:1职工信息的录入,2职工信息的显示3.用对象数组保存已输入的职工对象4.可以修改人员的基本信息5.可以通过编号或姓名进行人员查询
时间: 2023-06-18 18:06:56 浏览: 386
好的,下面是一个简单的实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Date {
public:
Date(int y, int m, int d) : year(y), month(m), day(d) {}
void display() {
cout << year << "-" << month << "-" << day << endl;
}
private:
int year;
int month;
int day;
};
class Employee {
public:
Employee(int id, string n, char s, Date b, string p) :
id(id), name(n), sex(s), birth(b), position(p) {}
void display() {
cout << "编号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "性别:" << sex << endl;
cout << "出生日期:";
birth.display();
cout << "职务:" << position << endl;
}
friend void modify(Employee& e);
friend class ManagementSystem;
private:
int id;
string name;
char sex;
Date birth;
string position;
};
void modify(Employee& e) {
cout << "请输入修改后的信息:" << endl;
cout << "编号:";
cin >> e.id;
cout << "姓名:";
cin >> e.name;
cout << "性别:";
cin >> e.sex;
cout << "出生日期(格式为yyyy-mm-dd):";
int year, month, day;
char dash;
cin >> year >> dash >> month >> dash >> day;
Date new_birth(year, month, day);
e.birth = new_birth;
cout << "职务:";
cin >> e.position;
}
class ManagementSystem {
public:
ManagementSystem() : num(0) {}
void input() {
cout << "请输入员工信息:" << endl;
int id;
string name;
char sex;
cout << "编号:";
cin >> id;
cout << "姓名:";
cin >> name;
cout << "性别:";
cin >> sex;
cout << "出生日期(格式为yyyy-mm-dd):";
int year, month, day;
char dash;
cin >> year >> dash >> month >> dash >> day;
Date birth(year, month, day);
string position;
cout << "职务:";
cin >> position;
Employee e(id, name, sex, birth, position);
employees[num++] = e;
}
void displayById(int id) {
for (int i = 0; i < num; i++) {
if (employees[i].id == id) {
employees[i].display();
return;
}
}
cout << "未找到该编号对应的员工" << endl;
}
void displayByName(string name) {
for (int i = 0; i < num; i++) {
if (employees[i].name == name) {
employees[i].display();
return;
}
}
cout << "未找到该姓名对应的员工" << endl;
}
void modifyById(int id) {
for (int i = 0; i < num; i++) {
if (employees[i].id == id) {
modify(employees[i]);
return;
}
}
cout << "未找到该编号对应的员工" << endl;
}
void modifyByName(string name) {
for (int i = 0; i < num; i++) {
if (employees[i].name == name) {
modify(employees[i]);
return;
}
}
cout << "未找到该姓名对应的员工" << endl;
}
private:
Employee employees[100];
int num;
};
int main() {
ManagementSystem ms;
while (true) {
cout << "请选择操作:" << endl;
cout << "1.录入员工信息" << endl;
cout << "2.查询员工信息(按编号)" << endl;
cout << "3.查询员工信息(按姓名)" << endl;
cout << "4.修改员工信息(按编号)" << endl;
cout << "5.修改员工信息(按姓名)" << endl;
cout << "6.退出程序" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
ms.input();
break;
case 2:
int id;
cout << "请输入编号:";
cin >> id;
ms.displayById(id);
break;
case 3:
string name;
cout << "请输入姓名:";
cin >> name;
ms.displayByName(name);
break;
case 4:
int modify_id;
cout << "请输入编号:";
cin >> modify_id;
ms.modifyById(modify_id);
break;
case 5:
string modify_name;
cout << "请输入姓名:";
cin >> modify_name;
ms.modifyByName(modify_name);
break;
case 6:
return 0;
default:
cout << "输入错误,请重新选择" << endl;
}
}
return 0;
}
```
注意,为了方便起见,这里的员工信息只保存在了程序运行期间,如果程序结束后再次运行,之前录入的员工信息就会丢失。如果需要实现永久保存员工信息的功能,可以将员工信息写入文件中,或者使用数据库等技术。
阅读全文
相关推荐
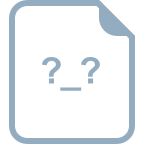














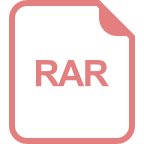

