nth_element
时间: 2023-11-30 17:03:10 浏览: 30
The `nth_element` algorithm is a function in the C++ Standard Template Library (STL) that is used to rearrange elements in a container such that the nth element is in its final sorted position, while all the other elements are unordered.
The syntax for using `nth_element` is:
```c++
nth_element(start_iterator, nth_element_iterator, end_iterator);
```
where `start_iterator` and `end_iterator` are the iterators defining the range of elements to be sorted, and `nth_element_iterator` is an iterator pointing to the nth element.
For example, consider the following code:
```c++
#include <iostream>
#include <algorithm>
#include <vector>
int main()
{
std::vector<int> vec = { 5, 2, 9, 1, 7, 3, 8, 6, 4 };
std::nth_element(vec.begin(), vec.begin() + 4, vec.end());
std::cout << "The fifth element is " << vec[4] << std::endl;
return 0;
}
```
In this example, `nth_element` is used to rearrange the elements in the `vec` vector such that the fifth element (i.e., `vec[4]`) is in its final sorted position. The output of the program will be:
```
The fifth element is 5
```
Note that the other elements in the vector are not sorted, but only the fifth element is guaranteed to be in its final position.
相关推荐
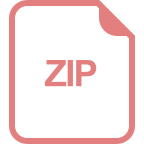
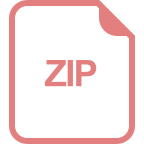
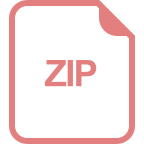














