请用golang实现布隆过滤器
时间: 2023-03-22 11:02:17 浏览: 183
好的,以下是用Golang实现布隆过滤器的示例代码:
```go
package main
import (
"bufio"
"fmt"
"hash/fnv"
"os"
)
type BloomFilter struct {
bitset []bool
k uint64
}
func NewBloomFilter(m uint64, k uint64) *BloomFilter {
return &BloomFilter{make([]bool, m), k}
}
func (bf *BloomFilter) Add(s string) {
for i := uint64(0); i < bf.k; i++ {
h := fnv.New64a()
h.Write([]byte(s))
index := h.Sum64() % uint64(len(bf.bitset))
bf.bitset[index] = true
}
}
func (bf *BloomFilter) Contains(s string) bool {
for i := uint64(0); i < bf.k; i++ {
h := fnv.New64a()
h.Write([]byte(s))
index := h.Sum64() % uint64(len(bf.bitset))
if !bf.bitset[index] {
return false
}
}
return true
}
func main() {
bf := NewBloomFilter(10000, 3)
// Add some strings to the Bloom filter
bf.Add("apple")
bf.Add("banana")
bf.Add("orange")
// Check if some strings are in the Bloom filter
fmt.Println(bf.Contains("apple")) // true
fmt.Println(bf.Contains("banana")) // true
fmt.Println(bf.Contains("orange")) // true
fmt.Println(bf.Contains("grape")) // false
fmt.Println(bf.Contains("peach")) // false
fmt.Println(bf.Contains("pineapple")) // false
// Read some strings from a file and check if they are in the Bloom filter
file, _ := os.Open("words.txt")
defer file.Close()
scanner := bufio.NewScanner(file)
for scanner.Scan() {
word := scanner.Text()
if bf.Contains(word) {
fmt.Printf("%s may be in the set\n", word)
}
}
}
```
以上是一个简单的布隆过滤器实现,它使用了FNV-1a哈希算法来计算字符串的哈希值,并将哈希值映射到一个布隆过滤器的位数组中,如果位数组中的所有位都被置位了,那么就认为这个字符串可能在集合中。注意,这个实现中的位数组大小是固定的,如果集合中的元素数量超过了位数组的大小,那么误判率就会增加。
阅读全文
相关推荐
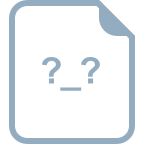

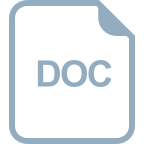
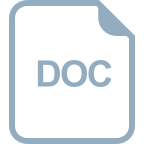
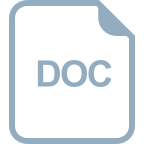
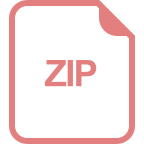
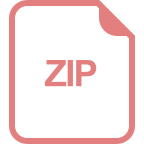
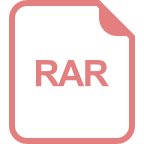
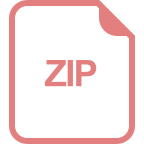
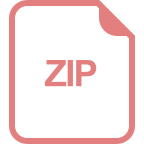
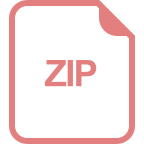
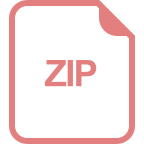
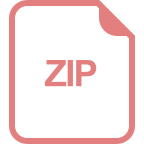
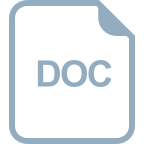
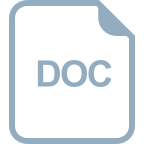
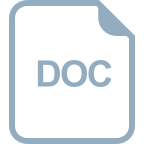
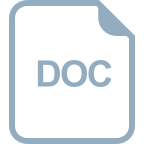