用c++编写挖方填方代码,使用dem数据
时间: 2024-02-13 07:00:27 浏览: 33
好的,挖方填方是一种常见的土木工程计算,可以使用DEM(数字高程模型)数据进行计算。下面是一个简单的C++程序示例,用于计算给定DEM数据中的挖方和填方量:
```c++
#include <iostream>
#include <fstream>
#include <cmath>
using namespace std;
const double PI = 3.14159265358979323846;
// 计算面积
double calcArea(double x1, double y1, double x2, double y2, double x3, double y3) {
return 0.5 * abs((x1 - x3) * (y2 - y3) - (x2 - x3) * (y1 - y3));
}
// 计算三角形的高
double calcHeight(double x1, double y1, double x2, double y2, double x3, double y3) {
double area = calcArea(x1, y1, x2, y2, x3, y3);
double edge = sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
return 2 * area / edge;
}
// 计算挖方和填方量
void calcVolume(double** data, int rows, int cols, double height) {
double volume = 0;
for (int i = 0; i < rows - 1; i++) {
for (int j = 0; j < cols - 1; j++) {
double x1 = j, y1 = i, z1 = data[i][j];
double x2 = j + 1, y2 = i, z2 = data[i][j + 1];
double x3 = j, y3 = i + 1, z3 = data[i + 1][j];
double x4 = j + 1, y4 = i + 1, z4 = data[i + 1][j + 1];
double h1 = max(0.0, height - z1);
double h2 = max(0.0, height - z2);
double h3 = max(0.0, height - z3);
double h4 = max(0.0, height - z4);
double v1 = h1 * calcArea(x1, y1, x2, y2, x3, y3);
double v2 = h2 * calcArea(x2, y2, x4, y4, x3, y3);
double v3 = h3 * calcArea(x1, y1, x3, y3, x4, y4);
double v4 = h4 * calcArea(x2, y2, x4, y4, x1, y1);
volume += (v1 + v2 + v3 + v4) / 4;
}
}
if (volume > 0) {
cout << "填方量: " << volume << endl;
}
else {
cout << "挖方量: " << -volume << endl;
}
}
int main() {
ifstream fin("dem.txt");
int rows, cols;
double** data;
fin >> rows >> cols;
data = new double*[rows];
for (int i = 0; i < rows; i++) {
data[i] = new double[cols];
for (int j = 0; j < cols; j++) {
fin >> data[i][j];
}
}
fin.close();
double height;
cout << "请输入高度: ";
cin >> height;
calcVolume(data, rows, cols, height);
for (int i = 0; i < rows; i++) {
delete[] data[i];
}
delete[] data;
return 0;
}
```
以上代码读取了一个名为“dem.txt”的DEM数据文件,该文件第一行包含行数和列数,接下来的每一行包含一系列高程数据。程序提示用户输入高度,然后计算挖方和填方量。
相关推荐
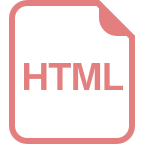
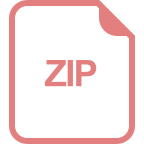
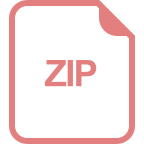














