JpaRepository 根据时间段查询数据
时间: 2024-05-16 14:17:06 浏览: 9
可以使用 Spring Data JPA 提供的 `Between` 关键字进行时间段查询。假设实体类为 `Entity`,需要根据时间段查询 `create_time` 字段:
```java
@Repository
public interface EntityRepository extends JpaRepository<Entity, Long> {
List<Entity> findByCreateTimeBetween(Date startTime, Date endTime);
}
```
其中 `findByCreateTimeBetween` 方法会自动解析方法名称,将 `Between` 后缀的参数作为时间段查询的起始和结束时间。可以通过传入 `java.util.Date` 类型的参数来指定时间段。
例如,以下代码会查询 2022 年 1 月 1 日到 2022 年 1 月 31 日之间的数据:
```java
Date startTime = new SimpleDateFormat("yyyy-MM-dd").parse("2022-01-01");
Date endTime = new SimpleDateFormat("yyyy-MM-dd").parse("2022-01-31");
List<Entity> result = entityRepository.findByCreateTimeBetween(startTime, endTime);
```
相关问题
JpaRepository 根据时间段查询数据,startTime:2023-05-05,endTime:2023-05-05
你可以使用 `JpaRepository` 提供的 `between` 关键字来查询时间段内的数据。具体操作示例如下:
```java
import java.util.Date;
import org.springframework.data.jpa.repository.JpaRepository;
public interface YourRepository extends JpaRepository<YourEntity, Long> {
List<YourEntity> findByCreateTimeBetween(Date startTime, Date endTime);
}
```
其中,`YourEntity` 是你的实体类,`createTime` 是你想要查询的时间字段。在方法名中使用 `Between` 关键字并传入起始时间和结束时间参数即可。你可以将 `startTime` 和 `endTime` 分别设置为 `2023-05-05` 的起始时间和结束时间进行查询。
JpaRepository 根据时间段查询今天的数据
您可以使用 `JpaRepository` 中的 `Between` 关键字来查询今天的数据。假设您的实体类为 `MyEntity`,其中有一个名为 `timestamp` 的字段表示时间戳,您可以在 `MyEntityRepository` 接口中添加一个方法:
```java
public interface MyEntityRepository extends JpaRepository<MyEntity, Long> {
List<MyEntity> findByTimestampBetween(LocalDateTime start, LocalDateTime end);
}
```
然后在您的代码中调用该方法,传入今天的起始时间和结束时间即可查询今天的数据:
```java
LocalDateTime todayStart = LocalDateTime.of(LocalDate.now(), LocalTime.MIN);
LocalDateTime todayEnd = LocalDateTime.of(LocalDate.now(), LocalTime.MAX);
List<MyEntity> todayEntities = myEntityRepository.findByTimestampBetween(todayStart, todayEnd);
```
这样就可以查询到今天的数据了。注意,时间的精度应该与您存储的时间戳字段相同。如果您的时间戳字段精确到毫秒,则应该使用 `Instant` 类型代替 `LocalDateTime` 类型。
相关推荐
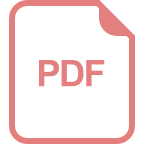
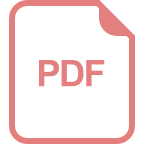







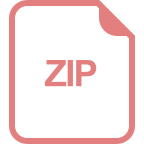
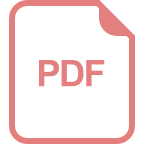
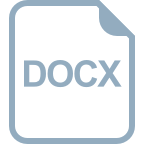
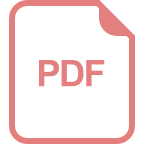