opencv视频区域反色python
时间: 2024-12-27 09:21:37 浏览: 2
### 使用 Python 和 OpenCV 对视频特定区域进行反色处理
为了实现对视频中的特定区域应用反色效果,可以按照以下方式编写代码。此方法涉及读取每一帧图像并对其指定部分执行操作。
#### 导入必要的库
首先导入所需的库,包括 `cv2` 用于计算机视觉任务以及 `numpy` 来辅助数组运算:
```python
import cv2
import numpy as np
```
#### 定义感兴趣区 (ROI)
定义一个函数来设置感兴趣的矩形区域坐标(x,y,w,h),其中 w 表示宽度而 h 是高度:
```python
def set_roi(frame, rect):
"""Set Region Of Interest based on given rectangle coordinates."""
x, y, width, height = rect
roi = frame[y:y+height, x:x+width]
return roi
```
#### 应用反色变换
创建另一个函数负责反转颜色值;这里采用简单的方式——减去当前像素强度与最大可能亮度之间的差额(即255对于8位灰度级而言):
```python
def invert_colors(image):
"""Invert colors of the input image."""
inverted_image = cv2.bitwise_not(image) # or use: 255 - image for grayscale images
return inverted_image
```
#### 主循环逻辑
最后构建主程序结构,打开摄像头获取实时画面,并持续更新显示直至按下 'q' 键退出:
```python
cap = cv2.VideoCapture(0)
while cap.isOpened():
ret, frame = cap.read()
if not ret:
break
# Define your ROI here; e.g., a square at center with side length=100 pixels.
rect = [(frame.shape[1]-100)//2, (frame.shape[0]-100)//2, 100, 100]
# Extract and process only within this area.
roi = set_roi(frame, rect)
processed_roi = invert_colors(roi)
# Place back into original position after processing.
frame[rect[1]:rect[1]+rect[3], rect[0]:rect[0]+rect[2]] = processed_roi
cv2.imshow('Frame', frame)
key = cv2.waitKey(1) & 0xFF
if key == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
上述脚本展示了如何捕获来自默认设备的直播流并将选定框内的所有内容转换成负片形式[^1]。
阅读全文
相关推荐
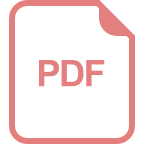
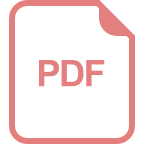















