Linux C++ 创建线程
时间: 2024-04-01 09:30:11 浏览: 163
在Linux C++中,可以使用pthread库来创建线程。下面是创建线程的基本步骤:
1. 包含头文件:首先需要包含pthread.h头文件,该头文件包含了线程相关的函数和数据类型。
2. 定义线程函数:创建线程时需要指定一个函数作为线程的入口点。该函数将在新线程中执行。函数的返回类型必须为void*,参数类型可以是任意类型。
3. 创建线程:使用pthread_create函数来创建线程。该函数接受四个参数:线程标识符、线程属性、线程函数、传递给线程函数的参数。
4. 等待线程结束:如果需要等待线程结束并获取其返回值,可以使用pthread_join函数。该函数接受两个参数:线程标识符和指向返回值的指针。
下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <pthread.h>
// 线程函数
void* threadFunc(void* arg) {
int threadId = *(int*)arg;
std::cout << "Hello from thread " << threadId << std::endl;
pthread_exit(NULL);
}
int main() {
pthread_t thread;
int threadId = 1;
// 创建线程
int ret = pthread_create(&thread, NULL, threadFunc, &threadId);
if (ret != 0) {
std::cerr << "Failed to create thread" << std::endl;
return 1;
}
// 等待线程结束
ret = pthread_join(thread, NULL);
if (ret != 0) {
std::cerr << "Failed to join thread" << std::endl;
return 1;
}
return 0;
}
```
阅读全文
相关推荐
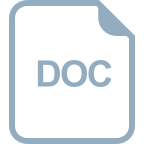
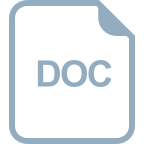
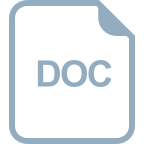
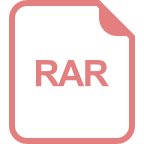
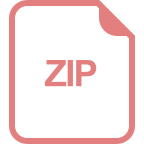
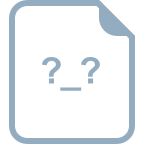
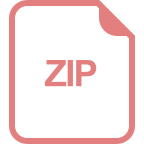
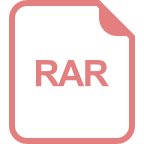
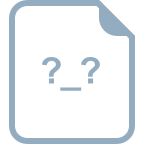
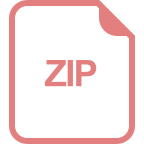
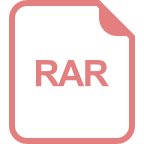
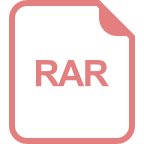
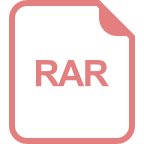
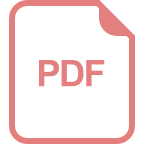
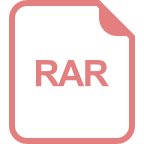
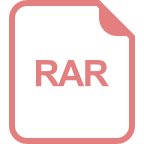
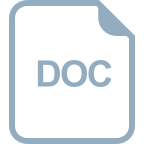
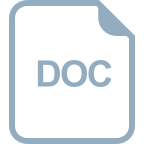
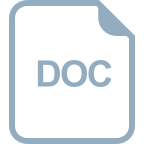