利用栈判断符号是否匹配,c语言来实现
时间: 2024-10-25 15:05:04 浏览: 30
在C语言中,我们可以使用栈数据结构来判断括号的符号是否匹配。括号主要有圆括号 '(', ')', 方括号 '[', ']', 和花括号 '{', '}'. 我们遍历输入字符串,对于每一对符号,按照特定规则检查它们是否能配对:
1. 如果遇到左括号 ('(', '[', 或 '{'),将其压入栈中。
2. 如果遇到右括号 (')', ']', 或 '}'),检查栈顶元素是否是对应的左括号。如果是,则弹出栈顶元素;如果不是,说明括号不匹配,返回false。
3. 遍历结束后,如果栈为空,说明所有括号都已配对,返回true;否则,返回false。
这里是一个简单的示例代码片段:
```c
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
bool is_valid_parentheses(char* str) {
stack_t* s = (stack_t*)malloc(sizeof(stack_t));
stack_init(s);
for(int i = 0; str[i] != '\0'; i++) {
if(str[i] == '(' || str[i] == '[' || str[i] == '{')
stack_push(s, str[i]);
else if(str[i] == ')' && !stack_is_empty(s)) {
char top = stack_pop(s);
if(top != '(')
return false;
}
else if(str[i] == ']' && !stack_is_empty(s)) {
char top = stack_pop(s);
if(top != '[')
return false;
}
else if(str[i] == '}' && !stack_is_empty(s)) {
char top = stack_pop(s);
if(top != '{')
return false;
}
}
// 如果遍历完字符串后栈还非空,表示还有未配对的左括号
if(!stack_is_empty(s))
return false;
free(s);
return true;
}
// 假设stack_t是一个自定义的栈结构,包含push(), pop()和is_empty()方法
typedef struct stack_t {
// 省略...
} stack_t;
// 假设stack_init(), stack_push(), stack_pop(), stack_is_empty()等函数已实现
int main() {
char str[] = "({[()]}())";
bool result = is_valid_parentheses(str);
printf("%s 的括号匹配吗? %s\n", str, result ? "是" : "否");
return 0;
}
```
运行上述代码后,会输出 `({[()]}()) 的括号匹配吗? 是`。
阅读全文
相关推荐
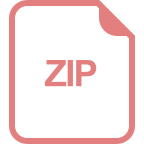
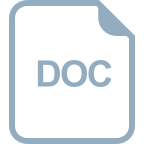
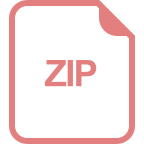
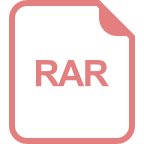
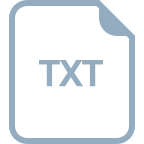
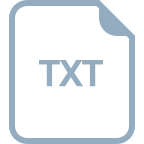
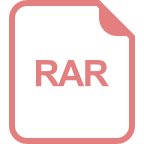
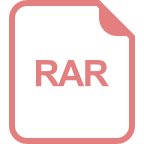
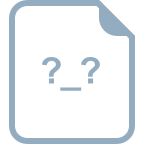
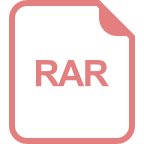
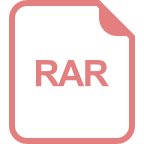
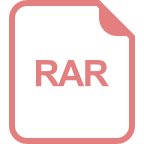
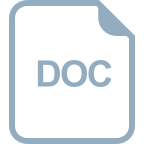
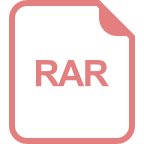
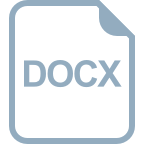
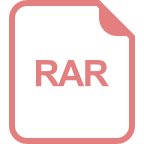
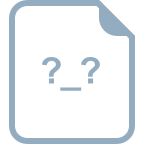
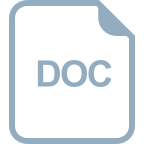
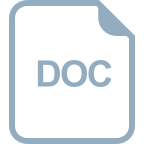