页面置换算法(最佳置换算法,先进先出算法,最近最久未使用算法,最少使用算法,至少完成其中任意两种算法的设计,并计算缺页率。)运行代码
时间: 2024-12-17 19:09:22 浏览: 8
页面置换算法是在操作系统内存管理中,当物理内存不足时,为了释放空间给新分配的页面而选择哪些页面需要淘汰到外存的一种策略。常见的页面置换算法有:
1. **最佳置换算法(Optimal Replacement Algorithm, ORA)**:这是理想化的算法,它总是将当前最长时间以来未使用的页面替换出去。在现实中难以实现,因为它需要预测未来的访问模式。
2. **先进先出算法(First-In-First-Out, FIFO)**:就像队列一样,最先进入内存的页面会被最先淘汰。这简单直观,但并不考虑近期的访问情况。
3. **最近最久未使用算法(Least Recently Used, LRU)**:它倾向于淘汰最后一次访问时间最久的页面。这种算法可以较好地追踪活跃页面,但在硬件支持下可能复杂。
4. **最少使用算法(Least Frequently Used, LFU)**:基于每个页面被访问的频率来决策,频率最低的页面被淘汰。这通常依赖于额外的数据结构来跟踪访问计数。
设计一个简单的LRU算法示例(这里假设Python语言):
```python
import collections
class LRU_Cache:
def __init__(self, capacity):
self.cache = collections.OrderedDict()
self.capacity = capacity
def get(self, key):
if key in self.cache:
# Move accessed item to the end of the ordered dictionary
self.cache.move_to_end(key)
return self.cache[key]
else:
return -1
def put(self, key, value):
if key in self.cache:
self.cache.pop(key) # Remove old entry before updating
elif len(self.cache) >= self.capacity:
self.cache.popitem(last=False) # Remove least recently used
self.cache[key] = value
# 示例使用
cache = LRU_Cache(2)
cache.put(1, 1)
cache.put(2, 2)
print(cache.get(1)) # 返回1
cache.put(3, 3) # 由于容量限制,会替换掉1(LRU)
print(cache.get(2)) # 返回2
print(cache.get(3)) # 返回3
```
至于计算缺页率,这个过程通常是基于理论模型的,比如平均访问时间(Average Access Time, AAT),在实际环境中很难直接获取准确值,因为涉及到操作系统内部细节和随机因素。一般通过模拟或者统计分析来近似估计。如果你需要进一步了解如何计算,我们可以讨论一下基本思路。
阅读全文
相关推荐
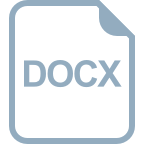
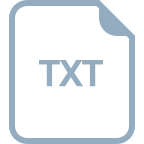

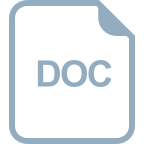
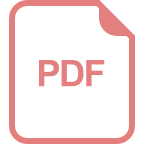
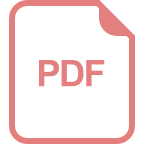
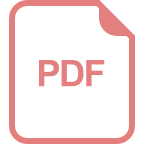
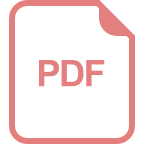
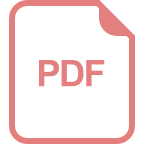
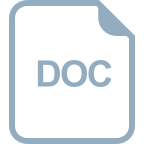
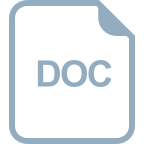
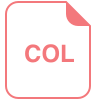
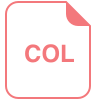
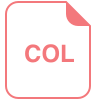
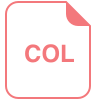

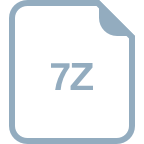