c 语言实现inotify监控某个文件夹,示例
时间: 2024-12-10 14:37:50 浏览: 11
在C语言中,实现inotify监控某个文件夹通常会利用`libinotify`库,这是一个第三方库,可以方便地操作Linux的inotify机制。以下是一个简单的示例,展示了如何使用`libinotify`来监视指定目录及其子目录的变化:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <fcntl.h>
#include <sys/inotify.h>
#define MAX_EVENTS 100
#define INOTIFY_EVENT_SIZE (sizeof(struct inotify_event) + PATH_MAX)
typedef struct {
int wd; // watch descriptor
char path[PATH_MAX];
} Watch;
static void event_handler(int fd, uint32_t mask, uint32_t cookie, void *ptr)
{
struct inotify_event *event = ptr;
char pathname[PATH_MAX];
if (read(fd, event, sizeof(*event) + PATH_MAX - 1) != sizeof(*event) + PATH_MAX - 1) {
perror("Reading from inotify");
return;
}
strncpy(pathname, event->name, PATH_MAX);
printf("[INOTIFY] %s: %d\n", pathname, event->mask);
}
int main()
{
int fd, wd;
char dir[] = "/path/to/your/directory"; // 替换为你想要监控的目录路径
struct inotify_init_data init_data;
struct inotify_event event;
Watch watches[MAX_EVENTS];
// 初始化inotify
if ((fd = inotify_init1(IN_CLOEXEC)) == -1) {
perror("inotify_init1");
exit(EXIT_FAILURE);
}
// 设置初始化数据
init_data wd_count = 0;
init_data.mask = IN_ALL_EVENTS; // 监听所有的事件类型
if (inotify_add_watch(fd, dir, init_data.mask) == -1) {
perror("inotify_add_watch");
exit(EXIT_FAILURE);
}
// 主循环
for (wd = 0; wd < MAX_EVENTS; wd++) {
while ((nbytes = read(fd, &event, sizeof(event))) > 0) {
watches[wd].wd = event.wd;
strncpy(watches[wd].path, event.pathname, PATH_MAX);
event_handler(fd, event.mask, event.cookie, &event);
}
if (nbytes == -1 && errno == EAGAIN) { // 如果发生EAGAIN错误,继续读取
continue;
} else if (nbytes == -1) {
perror("Reading from inotify");
break;
}
}
close(fd);
return 0;
}
```
注意:这个示例需要链接`libinotify.so`库,如果你的项目中没有包含,需要先安装它。另外,在实际生产环境中,建议使用更完善的错误处理和资源管理。
阅读全文
相关推荐
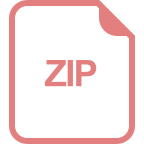
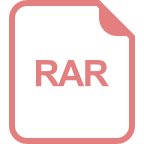
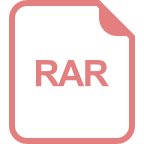

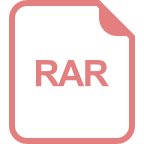
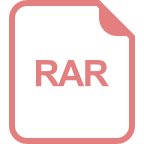
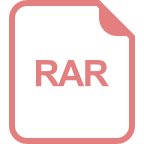
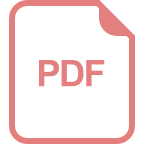
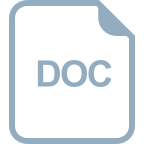
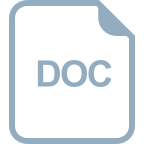
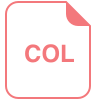
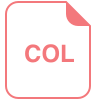
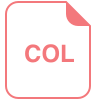
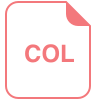
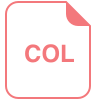
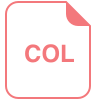


