抽象类Shape C++
时间: 2024-06-20 12:03:43 浏览: 16
在C++中,抽象类是指不能实例化对象的类,通常用于作为其他派生类的基类。抽象类通过纯虚函数(即没有实现的虚函数)来定义接口,使得派生类必须实现这些接口才能被实例化。
Shape可以作为一个抽象类的例子。它定义了几何图形的基本属性和操作,但没有具体的实现。例如,Shape定义了计算面积和周长的纯虚函数,但并没有给出具体的计算方法。这样,派生类可以继承Shape,并实现自己的计算方法来计算面积和周长。
以下是Shape的示例代码:
```
class Shape {
public:
virtual double area() const = 0; // 计算面积的纯虚函数
virtual double perimeter() const = 0; // 计算周长的纯虚函数
virtual ~Shape() {} // 虚析构函数,确保派生类释放资源
};
```
相关问题
抽象类C++PTA程序
抽象类是指不能被实例化,只能被用作父类的类。在 C++ 中,抽象类可以用纯虚函数来定义,而纯虚函数是没有实现的虚函数,它的声明以及纯虚函数的数量由具体的子类来实现。
在 PTA 程序中,抽象类常常用于定义一些具有共同特征的类。例如,可以定义一个 Shape 类作为所有形状的父类,其中包含一个纯虚函数计算面积。然后可以针对不同的形状(如圆形、矩形等)定义具体的子类,并实现相应的计算面积函数。这样可以方便地管理和操作不同形状的对象。
另外需要注意的是,抽象类不能直接实例化,但可以被指向子类对象的指针或引用所使用。也就是说,在使用抽象类时需要用具体的子类对象来进行操作。
C++抽象类
C++中抽象类是指包含纯虚函数的类,它不能被实例化,只能作为其他类的基类来派生新的类。纯虚函数是在基类中声明的虚函数,但没有给出实现,派生类必须重写这些纯虚函数才能被实例化。
抽象类的声明方式为在类名后面加上 "= 0" 的纯虚函数,例如:
```c++
class Shape {
public:
virtual void draw() = 0; // 纯虚函数
};
```
这里声明了一个名为Shape的抽象类,它包含一个纯虚函数draw(),没有函数体。
派生类可以继承抽象类,但必须实现所有的纯虚函数才能被实例化。例如:
```c++
class Circle : public Shape {
public:
void draw() {
// 实现绘制圆形的代码
}
};
```
这里声明了一个名为Circle的类,它继承自Shape抽象类,并且实现了draw()函数。
抽象类的目的是为了让继承它的类实现某些基本的功能,而不用在每个派生类中都重复实现。
相关推荐
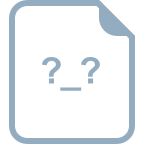
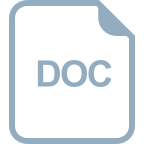












