用python代码做一个推箱子的游戏可以随机生成关卡
时间: 2024-02-01 22:04:32 浏览: 144
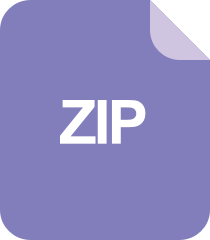
基于 Python 制作推箱子小游戏【100011857】
好的,推箱子游戏可以用Python的Pygame库来实现,关卡的生成可以使用随机算法来实现。
首先,我们需要安装Pygame库。可以使用以下命令安装:
```python
pip install pygame
```
接下来,我们可以开始写代码了。以下是一个简单的推箱子游戏的代码示例:
```python
import pygame
import random
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
BLUE = (50, 153, 213)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 设置屏幕大小
SCREEN_WIDTH = 800
SCREEN_HEIGHT = 600
# 定义方块大小
BLOCK_SIZE = 50
# 定义关卡地图
level = [
"##########",
"# #",
"# $ # #",
"# @ # #",
"# . # #",
"# $ # #",
"# #",
"##########"
]
# 定义游戏对象类
class GameObject:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
def draw(self, screen):
pygame.draw.rect(screen, self.color, [self.x, self.y, BLOCK_SIZE, BLOCK_SIZE])
# 定义箱子类
class Box(GameObject):
def move(self, dx, dy):
self.x += dx
self.y += dy
# 初始化游戏
def init_game():
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode([SCREEN_WIDTH, SCREEN_HEIGHT])
pygame.display.set_caption("推箱子游戏")
# 加载图像
player_image = pygame.image.load("player.png").convert()
box_image = pygame.image.load("box.png").convert()
# 定义游戏对象
player = GameObject(0, 0, GREEN)
boxes = []
# 遍历关卡地图,生成游戏对象
for row in range(len(level)):
for col in range(len(level[row])):
if level[row][col] == "#":
# 墙壁
obj = GameObject(col * BLOCK_SIZE, row * BLOCK_SIZE, BLUE)
elif level[row][col] == "$":
# 箱子
obj = Box(col * BLOCK_SIZE, row * BLOCK_SIZE, WHITE)
boxes.append(obj)
elif level[row][col] == "@":
# 玩家
player.x = col * BLOCK_SIZE
player.y = row * BLOCK_SIZE
obj = GameObject(col * BLOCK_SIZE, row * BLOCK_SIZE, BLACK)
elif level[row][col] == ".":
# 目标点
obj = GameObject(col * BLOCK_SIZE, row * BLOCK_SIZE, RED)
obj.draw(screen)
# 绘制玩家和箱子
player.draw(screen)
for box in boxes:
box.draw(screen)
pygame.display.flip()
return screen, player, boxes, player_image, box_image
# 处理游戏事件
def handle_events(player, boxes):
for event in pygame.event.get():
if event.type == pygame.QUIT:
return True
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
dx, dy = -BLOCK_SIZE, 0
elif event.key == pygame.K_RIGHT:
dx, dy = BLOCK_SIZE, 0
elif event.key == pygame.K_UP:
dx, dy = 0, -BLOCK_SIZE
elif event.key == pygame.K_DOWN:
dx, dy = 0, BLOCK_SIZE
else:
dx, dy = 0, 0
# 移动玩家和箱子
player.move(dx, dy)
for box in boxes:
if player.x == box.x and player.y == box.y:
box.move(dx, dy)
return False
# 更新游戏画面
def update_screen(screen, player, boxes, player_image, box_image):
# 绘制地图
for row in range(len(level)):
for col in range(len(level[row])):
if level[row][col] == "#":
# 墙壁
color = BLUE
elif level[row][col] == ".":
# 目标点
color = RED
else:
color = WHITE
pygame.draw.rect(screen, color, [col * BLOCK_SIZE, row * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE])
# 绘制玩家和箱子
screen.blit(player_image, [player.x, player.y])
for box in boxes:
screen.blit(box_image, [box.x, box.y])
pygame.display.flip()
# 随机生成关卡
def generate_level():
level = []
for row in range(10):
line = ""
for col in range(10):
if row == 0 or row == 9 or col == 0 or col == 9:
# 边界
line += "#"
else:
# 随机生成空地或箱子
if random.random() < 0.2:
line += "$"
else:
line += " "
level.append(line)
level[5] = level[5][:5] + "@" + level[5][6:]
level[4] = level[4][:4] + "." + level[4][5:]
level[6] = level[6][:4] + "." + level[6][5:]
return level
# 主函数
def main():
# 随机生成关卡
level = generate_level()
# 初始化游戏
screen, player, boxes, player_image, box_image = init_game()
# 循环处理游戏事件,直到退出游戏
while True:
if handle_events(player, boxes):
break
update_screen(screen, player, boxes, player_image, box_image)
# 关闭Pygame
pygame.quit()
if __name__ == "__main__":
main()
```
这个示例代码中,我们定义了一个关卡地图,并随机生成了一个新的关卡。在游戏中,玩家可以通过方向键来移动,移动时会同时移动箱子。更新游戏画面时,我们使用了两张图像来代表玩家和箱子。
阅读全文
相关推荐
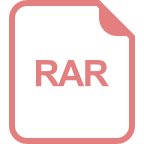
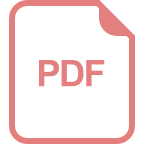
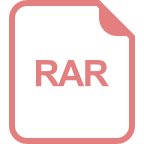
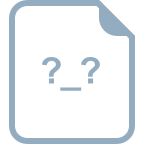
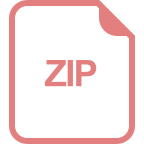
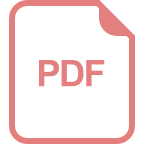
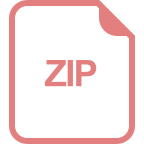
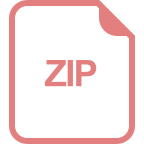
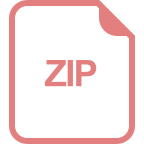
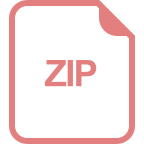
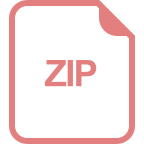
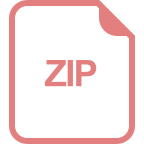