img_edge = sobel(img_gray, axis=0) + sobel(img_gray, axis=1)是什么意思
时间: 2024-03-31 22:35:15 浏览: 110
这行代码是在使用Sobel算子对灰度图像进行边缘检测。其中,img_gray是输入的灰度图像,sobel是Sobel算子函数,axis=0表示在x轴方向上进行Sobel算子运算,axis=1表示在y轴方向上进行Sobel算子运算。这行代码的作用是将x轴和y轴方向上的边缘检测结果相加,得到最终的边缘检测结果。
相关问题
将以下代码增加保存图像功能: import matplotlib.pyplot as plt import numpy as np from skimage import io, filters, measure, color from skimage.filters import threshold_otsu from skimage import io, color, filters img = io.imread('leaf.jpg') gray_img = color.rgb2gray(img) thresh = filters.threshold_otsu(gray_img) binary = gray_img > thresh edges = filters.sobel(binary) fig, axes = plt.subplots(nrows=1, ncols=2, figsize=(8, 3)) ax = axes.ravel() ax[0].imshow(img) ax[0].set_title("Original") ax[1].imshow(edges, cmap='gray') ax[1].set_title("Edges") for a in ax: a.axis('off') plt.tight_layout() plt.show()
以下是增加保存图像功能的代码:
```Python
import matplotlib.pyplot as plt
import numpy as np
from skimage import io, filters, measure, color
from skimage.filters import threshold_otsu
# 读取图像
img = io.imread('leaf.jpg')
gray_img = color.rgb2gray(img)
# 图像处理
thresh = filters.threshold_otsu(gray_img)
binary = gray_img > thresh
edges = filters.sobel(binary)
# 绘制图像
fig, axes = plt.subplots(nrows=1, ncols=2, figsize=(8, 3))
ax = axes.ravel()
ax[0].imshow(img)
ax[0].set_title("Original")
ax[1].imshow(edges, cmap='gray')
ax[1].set_title("Edges")
for a in ax:
a.axis('off')
# 保存图像
plt.savefig('edges.png')
plt.tight_layout()
plt.show()
```
在代码的最后,我们使用 `plt.savefig()` 函数将绘制的图像保存为文件,文件名为 "edges.png"。这样,在程序运行结束后,我们就可以在当前工作目录中找到保存的图像文件了。
import numpy as np import matplotlib.pyplot as plt from scipy import signal t = np.linspace(0, 2 * np.pi, 128, endpoint=False) x = np.sin(2 * t) print(x) kernel1 = np.array([[-1, 0, 1], [-2, 0, 2], [-1, 0, 1]]) kernel2 = np.array([[1, 2, 1], [0, 0, 0], [-1, -2, -1]]) result1 = signal.convolve2d(x.reshape(1, -1), kernel1, mode='same') result2 = signal.convolve2d(x.reshape(1, -1), kernel2, mode='same') fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].imshow(kernel1) axs[1].set_title('Kernel 1') axs[2].imshow(kernel2) axs[2].set_title('Kernel 2') fig.tight_layout() fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].plot(t, result1.flatten()) axs[1].set_title('Result of convolution with kernel 1') axs[2].plot(t, result2.flatten()) axs[2].set_title('Result of convolution with kernel 2') fig.tight_layout() plt.show() # from scipy.signal import pool import numpy as np def pool(signal, window_size, mode='max'): if mode == 'max': return np.max(signal.reshape(-1, window_size), axis=1) elif mode == 'min': return np.min(signal.reshape(-1, window_size), axis=1) elif mode == 'mean': return np.mean(signal.reshape(-1, window_size), axis=1) else: raise ValueError("Invalid mode. Please choose 'max', 'min', or 'mean'.") # 对卷积结果进行最大池化 pool_size = 2 result1_pooled = pool(result1, pool_size, 'max') result2_pooled = pool(result2, pool_size, 'max') # 可视化结果 fig, axs = plt.subplots(3, 1, figsize=(8, 8)) axs[0].plot(t, x) axs[0].set_title('Original signal') axs[1].plot(t, result1.flatten()) axs[1].set_title('Result of convolution with kernel 1') axs[2].plot(t[::2], result1_pooled.flatten()) axs[2].set_title('Result of max pooling after convolution with kernel 1') fig.tight_layout() plt.show()分析这段代码写出它每一步的用意和功能
这段代码主要实现了信号处理中的卷积和池化操作,并可视化其结果。
1. 导入numpy、matplotlib.pyplot和scipy.signal库。
2. 生成一个长度为128的正弦信号x。这里使用np.linspace()函数生成一个从0到2π的等差数列,再通过np.sin()函数将其转化为正弦信号。
3. 定义两个卷积核kernel1和kernel2,分别为Sobel算子的垂直和水平方向的卷积核。
4. 使用scipy.signal库的convolve2d()函数对信号x进行卷积操作,得到result1和result2分别为使用kernel1和kernel2卷积后的结果。
5. 使用matplotlib库的subplots()函数创建一个3行1列的子图,并绘制原始信号x、卷积核kernel1和卷积核kernel2。
6. 使用matplotlib库的subplots()函数创建一个3行1列的子图,并绘制原始信号x、卷积结果result1和卷积结果result2。
7. 使用自定义的pool()函数对卷积结果进行最大池化操作,得到result1_pooled和result2_pooled分别为使用kernel1和kernel2卷积后的结果经过最大池化后的结果。
8. 使用matplotlib库的subplots()函数创建一个3行1列的子图,并绘制原始信号x、卷积结果result1、经过最大池化后的结果result1_pooled,以及卷积结果result2、经过最大池化后的结果result2_pooled。
阅读全文
相关推荐
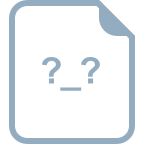
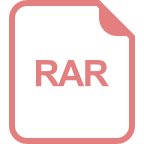
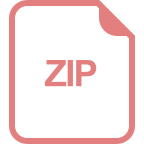
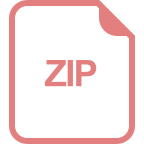
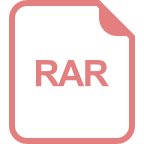
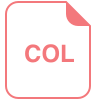








