请用vscode的opencv库,编写python代码实现以下功能,用户点击鼠标,利用摄像头抓拍一张图片,然后给抓拍到的图片添加高斯噪音,然后对图片进行均值滤波去噪,然后对图片进行一次sobel算子检测图片边缘,要求显示4张图片
时间: 2024-10-13 16:06:58 浏览: 40
要在VSCode中使用OpenCV库来实现这个功能,你需要安装`opencv-python`和`matplotlib`库。下面是一个简单的Python脚本示例,展示了如何完成这些步骤:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 初始化摄像头
cap = cv2.VideoCapture(0) # 使用默认摄像头,也可以指定摄像头ID
# 图像预处理函数
def add_noise_and_filter(img):
# 添加高斯噪声
noisy_img = img + np.random.normal(scale=50, size=img.shape)
# 对图像进行均值滤波去噪
blurred_img = cv2.blur(noisy_img, (5, 5))
return blurred_img
# 主循环
while True:
# 读取一帧
ret, frame = cap.read()
if not ret:
break
# 点击事件
click_pos = None
while click_pos is None:
cv2.imshow('Click to capture', frame)
_, click_pos = cv2.waitKeyEx(-1) & 0xFF # 等待用户点击
# 在点击位置截图
x, y = click_pos
cropped_img = frame[y:y+100, x:x+100] # 假设我们截取100x100像素区域
# 应用操作并显示结果
processed_img = add_noise_and_filter(cropped_img)
sobel_img = cv2.Sobel(processed_img, cv2.CV_8U, 1, 1, ksize=3) # 检测边缘
fig, axs = plt.subplots(nrows=2, ncols=2, figsize=(10, 10))
axs[0, 0].imshow(frame, cmap='gray')
axs[0, 0].set_title('Original Captured Image')
axs[0, 1].imshow(noisy_img, cmap='gray')
axs[0, 1].set_title('Noisy Image')
axs[1, 0].imshow(blurred_img, cmap='gray')
axs[1, 0].set_title('Blurred Image')
axs[1, 1].imshow(sobel_img, cmap='gray')
axs[1, 1].set_title('Edge Detection (Sobel)')
for ax in axs.flat:
ax.axis('off')
plt.tight_layout()
plt.show()
# 关闭摄像头
cap.release()
cv2.destroyAllWindows()
```
阅读全文
相关推荐
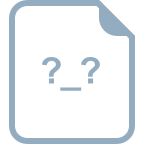
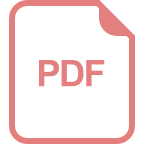
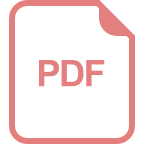




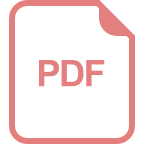
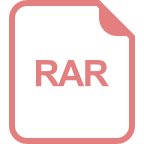
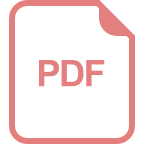
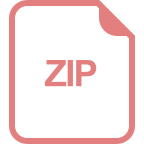





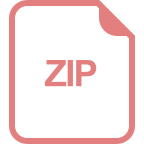
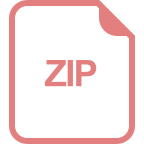