vue3如何实现页面背景由线条组成且可以随鼠标的移动而使背景移动
时间: 2024-02-24 12:58:07 浏览: 20
实现页面背景由线条组成的效果可以使用 SVG 和 Canvas 技术,而跟随鼠标移动的效果可以使用鼠标事件和 CSS transform 属性。
具体实现步骤如下:
1. 使用 SVG 或 Canvas 技术绘制线条背景,可以使用一些第三方库如 Three.js、D3.js 来完成。
2. 监听鼠标移动事件,获取鼠标位置。
3. 使用 CSS transform 属性移动背景,将背景移动的距离与鼠标位置相关联,可以使用 JavaScript 计算出移动距离。
下面是一个示例的代码,使用了 Three.js 绘制线条背景,并且使用了 Vue.js 3.0 的组件方式进行封装:
```html
<template>
<div class="background">
<canvas ref="canvas"></canvas>
</div>
</template>
<script>
import * as THREE from 'three';
export default {
mounted() {
// 创建 Three.js 场景
const scene = new THREE.Scene();
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
const renderer = new THREE.WebGLRenderer({ canvas: this.$refs.canvas });
// 创建线条背景
const material = new THREE.LineBasicMaterial({ color: 0xffffff });
const geometry = new THREE.BufferGeometry().setFromPoints([
new THREE.Vector3(-10, 0, 0),
new THREE.Vector3(0, 10, 0),
new THREE.Vector3(10, 0, 0),
]);
const line = new THREE.Line(geometry, material);
scene.add(line);
// 监听鼠标移动事件
window.addEventListener('mousemove', (event) => {
const x = event.clientX / window.innerWidth - 0.5;
const y = event.clientY / window.innerHeight - 0.5;
line.position.x = x * 10;
line.position.y = y * 10;
});
// 渲染场景
camera.position.z = 5;
function animate() {
requestAnimationFrame(animate);
renderer.render(scene, camera);
}
animate();
},
};
</script>
<style>
.background {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
overflow: hidden;
}
canvas {
width: 100%;
height: 100%;
}
</style>
```
上面的代码中,使用了 Three.js 创建了一个线条背景,然后监听了鼠标移动事件,根据鼠标位置计算出背景需要移动的距离,使用 CSS transform 属性进行移动,最后使用 requestAnimationFrame 函数来不断渲染场景。
相关推荐









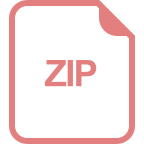