c++ 多级反馈队列算法
时间: 2023-07-26 22:13:38 浏览: 206
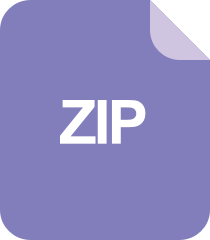
多级反馈队列调度算法(c++)
多级反馈队列算法是一种用于调度进程的算法,它将进程分为多个队列,并根据进程的优先级和执行时间的长度将进程放入不同的队列中。队列越高,进程的优先级越高,执行时间也越短。当一个进程在一个队列中执行了一段时间后,如果还没有完成,它将被移到更低优先级的队列中,以便给更高优先级的进程更多的机会。
以下是一个简单的 C++ 实现多级反馈队列算法的代码示例:
```c++
#include <iostream>
#include <queue>
using namespace std;
// 进程结构体
struct Process {
int id; // 进程 ID
int priority; // 进程优先级
int execution; // 执行时间
};
// 定义多级反馈队列
queue<Process> q1, q2, q3;
// 调度函数
void schedule() {
// 先处理高优先级队列 q1
if (!q1.empty()) {
Process p = q1.front();
q1.pop();
// 执行进程
cout << "Process " << p.id << " is running in q1." << endl;
// 如果还未执行完成,则放入下一个队列 q2
if (p.execution > 1) {
p.execution--;
p.priority++;
q2.push(p);
}
}
// 如果 q1 为空,则处理中优先级队列 q2
else if (!q2.empty()) {
Process p = q2.front();
q2.pop();
// 执行进程
cout << "Process " << p.id << " is running in q2." << endl;
// 如果还未执行完成,则放入下一个队列 q3
if (p.execution > 1) {
p.execution--;
p.priority++;
q3.push(p);
}
}
// 如果 q1 和 q2 都为空,则处理低优先级队列 q3
else if (!q3.empty()) {
Process p = q3.front();
q3.pop();
// 执行进程
cout << "Process " << p.id << " is running in q3." << endl;
// 如果还未执行完成,则放回队列 q3
if (p.execution > 1) {
p.execution--;
q3.push(p);
}
}
}
int main() {
// 将进程放入队列
q1.push({1, 3, 3});
q1.push({2, 1, 4});
q1.push({3, 2, 2});
q1.push({4, 4, 1});
// 调度进程
while (!q1.empty() || !q2.empty() || !q3.empty()) {
schedule();
}
return 0;
}
```
在这个示例中,我们定义了一个简单的进程结构体,包括进程 ID、优先级和执行时间。我们使用三个队列 q1、q2 和 q3 来模拟多级反馈队列,其中 q1 为高优先级队列,q2 为中优先级队列,q3 为低优先级队列。在调度函数中,我们首先处理 q1 中的进程,如果 q1 中没有进程,则处理 q2 中的进程,如果 q2 中也没有进程,则处理 q3 中的进程。在处理进程时,我们根据进程的优先级和执行时间将其放入不同的队列中,以模拟多级反馈队列算法的调度过程。
阅读全文
相关推荐
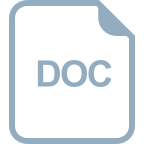
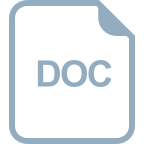
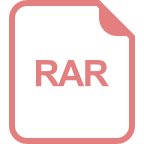
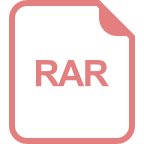
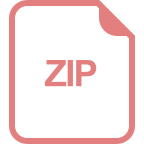
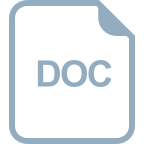
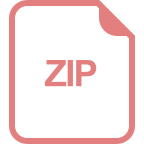





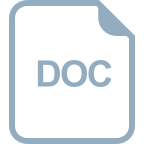
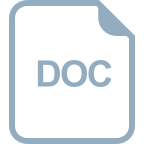
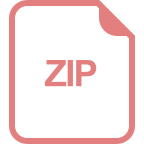