python的pandas选取特定的行
时间: 2023-09-26 18:11:04 浏览: 52
选取特定的行可以使用iloc和loc方法。
iloc方法:
- 通过行号选取,使用iloc方法,例如选取第2行到第4行的数据:
```python
import pandas as pd
df = pd.read_csv('data.csv')
df.iloc[1:4]
```
- 通过布尔索引选取,例如选取age列大于30的数据:
```python
df[df['age']>30]
```
loc方法:
- 通过行标签选取,使用loc方法,例如选取行标签为1、3、5的数据:
```python
df.loc[[1,3,5]]
```
- 通过行标签和列标签选取,例如选取行标签为1、3、5,列标签为name和age的数据:
```python
df.loc[[1,3,5],['name','age']]
```
相关问题
python选取excel特定行筛选行最大值
如果您只想选取Excel表格中的特定行进行筛选,可以在读取Excel文件后,使用DataFrame中的iloc函数选取指定的行。例如,如果要选取Excel表格中的第2行到第10行进行筛选,可以使用以下代码:
```python
import pandas as pd
df = pd.read_excel('your_file_path.xlsx', header=None, skiprows=1, nrows=9)
```
其中,header=None表示Excel表格中没有列名,skiprows=1表示跳过第一行(Excel表格中的列名),nrows=9表示选取Excel表格中的第2行到第10行(共9行)。
然后,您可以按照上述方法使用DataFrame中的max()和idxmax()函数进行筛选,例如:
```python
df['max_value'] = df.max(axis=1)
df['max_column'] = df.idxmax(axis=1)
filtered_df = df[df['max_value'] > some_value]
```
其中,some_value是您的筛选条件。需要注意的是,由于选取的是Excel表格中的特定行,因此在使用idxmax()函数时,需要将返回的列名加上选取的行号,例如:"B3"表示Excel表格中的第3行第2列。
pandas根据条件选取特定条件的行
好的,关于pandas根据条件选取特定条件的行,可以使用DataFrame的 loc 和 iloc 方法,配合布尔索引进行筛选。例如,可以使用 loc 方法选取符合某个条件(例如某一列的值等于某个特定值)的行,例如:
```python
import pandas as pd
df = pd.DataFrame([[1,2,3], [4,5,6], [7,8,9]], columns=["A", "B", "C"])
selected_rows = df.loc[df["A"] > 3]
print(selected_rows)
```
这个例子是选取了 DataFrame 中列 A 大于 3 的所有行。iloc 方法则是根据行号进行选取,例如:
```python
import pandas as pd
df = pd.DataFrame([[1,2,3], [4,5,6], [7,8,9]], columns=["A", "B", "C"])
selected_rows = df.iloc[[0, 2]]
print(selected_rows)
```
这个例子是选取了 DataFrame 中行号为 0 和 2 的行。
相关推荐
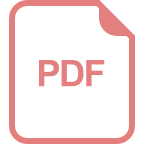
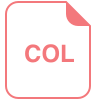
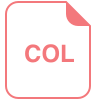
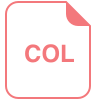
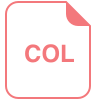
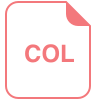







