请用C语言建立学生信息的线性表结构(链表),并在此基础上完成增、删、改、查等基本操作,代码以文档方式上交,同时需要运行结果
时间: 2024-09-22 09:04:18 浏览: 25
在C语言中,我们可以创建一个简单的链表结构来存储学生的个人信息。首先,我们需要定义一个结构体`Student`来保存学生的信息,比如学号、姓名和成绩。然后,我们再创建一个`Node`结构来代表链表中的每个节点,并包含指向下一个节点的指针。
```c
// 定义学生信息结构体
typedef struct {
int id;
char name[50];
float score;
} Student;
// 定义链表节点结构
typedef struct Node {
Student info; // 学生信息
struct Node* next; // 指向下一个节点的指针
} ListNode;
// 创建新节点
ListNode* createNode(Student s) {
ListNode* newNode = (ListNode*)malloc(sizeof(ListNode));
newNode->info = s;
newNode->next = NULL;
return newNode;
}
// 添加节点到链表
void addNode(ListNode** head, Student s) {
ListNode* newNode = createNode(s);
if (*head == NULL) {
*head = newNode;
} else {
ListNode* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
}
// 删除节点(这里仅删除id相同的第一个节点)
void deleteNode(ListNode** head, int id) {
if (*head == NULL) {
return;
}
if ((*head)->info.id == id) {
ListNode* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
ListNode* prev = *head;
ListNode* current = *head->next;
while (current != NULL && current->info.id != id) {
prev = current;
current = current->next;
}
if (current != NULL) {
prev->next = current->next;
free(current);
}
}
// 修改节点信息
void updateNode(ListNode** head, int id, char* newName, float newScore) {
ListNode* current = *head;
while (current != NULL) {
if (current->info.id == id) {
strncpy(current->info.name, newName, sizeof(current->info.name) - 1);
current->info.score = newScore;
break;
}
current = current->next;
}
}
// 查找节点(返回是否存在该id的学生)
int searchNode(ListNode* head, int id) {
ListNode* temp = head;
while (temp != NULL) {
if (temp->info.id == id) {
return 1; // 存在
}
temp = temp->next;
}
return 0; // 不存在
}
// 打印链表中的所有学生信息
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != NULL) {
printf("ID: %d, Name: %s, Score: %.2f\n", temp->info.id, temp->info.name, temp->info.score);
temp = temp->next;
}
}
```
为了运行这个链表,你需要将上述函数放入一个主函数中,并实例化链表。例如:
```c
int main() {
// 初始化链表
ListNode* head = NULL;
// 添加、修改、查找和删除操作...
// 打印链表
printList(head);
return 0;
}
```
相关推荐
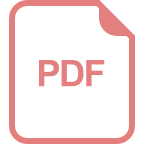
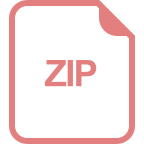














