能否提供一个详细的Python代码示例,展示如何使用特征脸方法进行人脸图像的预处理、主成分分析(PCA)、特征脸提取、人脸识别和特征向量的重构过程?同时,请给出相关的注意事项和可能产生的结果分析关键点。
时间: 2024-12-22 21:18:00 浏览: 3
当然可以。在Python中,我们可以使用sklearn库来进行PCA和特征脸(Eigenfaces)的实现。首先,确保已经安装了`numpy`, `matplotlib`, 和 `sklearn`库。这里是一个简化的步骤:
```python
import numpy as np
from sklearn.decomposition import PCA
from sklearn.datasets import fetch_olivetti_faces
from matplotlib import pyplot as plt
# 1. 数据预处理
# 下载并加载Olivetti人脸数据集(已包含归一化)
data = fetch_olivetti_faces()
X = data.images.reshape((len(data.images), -1)) / 255.0 # 归一化到[0,1]范围
# 2. 主成分分析 (PCA)
pca = PCA(n_components=2) # 我们只取前两个主成分
X_pca = pca.fit_transform(X)
# 3. 特征脸提取
eigenfaces = pca.components_.reshape(pca.n_components_, 64, 64) # 每张特征脸都是64x64像素
# 4. 人脸识别
def recognize_face(image, eigenfaces, pca):
image = image.reshape(1, -1) / 255.0 # 归一化
feature_vector = pca.transform(image) # 使用PCA将图像转换成特征向量
min_distance = np.inf
closest_eigenface_index = None
for i, eigenface in enumerate(eigenfaces):
distance = np.linalg.norm(feature_vector - eigenface)
if distance < min_distance:
min_distance = distance
closest_eigenface_index = i
return closest_eigenface_index
# 5. 示例人脸识别并可视化
image_to_recognize = X[0]
predicted_index = recognize_face(image_to_recognize, eigenfaces, pca)
reconstructed_image = eigenfaces[predicted_index].reshape(64, 64)
plt.subplot(1, 2, 1)
plt.imshow(image_to_recognize.reshape(64, 64), cmap='gray')
plt.title('Original Image')
plt.subplot(1, 2, 2)
plt.imshow(reconstructed_image, cmap='gray')
plt.title('Reconstructed Image')
# 注意事项:
# a. 预处理阶段的归一化很重要,避免因为像素值差异影响后续分析。
# b. 主成分的数量需适当选择,过多可能导致信息丢失,过少可能识别效果不佳。
# c. 在人脸识别时,特征向量的距离计算可以选择欧氏距离或其他相似度指标。
# 结果分析:
# - 最近邻匹配的结果可能是正确的,也可能不是,取决于训练数据和测试样本之间的分布。
# - 可通过调整PCA的成分数观察对识别效果的影响,以及图像重构的质量。
阅读全文
相关推荐
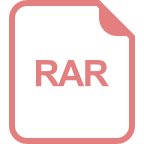
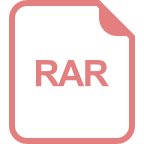
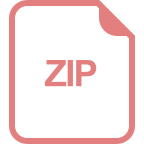















