public class ThreadHomework { public static void main(String[] args) { T1 t1 = new T1(); T2 t2 = new T2(t1); Thread th1 = new Thread(t1); Thread th2 = new Thread(t2); th1.start(); th2.start(); } } class T1 implements Runnable { @Override public void run() { for (int i = 1; i <= 10; i++) { synchronized (this) { System.out.println("hello,world " + i); notify(); try { wait(); } catch (InterruptedException e) { e.printStackTrace(); } } } synchronized (this) { notify(); } } } class T2 implements Runnable { private T1 t1; public T2(T1 t1) { this.t1 = t1; } @Override public void run() { for (int i = 1; i <= 5; i++) { synchronized (t1) { try { t1.wait(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("hi " + i); t1.notify(); } } } } 编译错误. java:5:错误:类T2中的构造函数T2不能应用于给定的类型; T2 T2=新的T2(); ^ 要求:T1 找到:没有参数 原因:实际论证列表和形式论证列表的长度不同 1个错误, 进一步修改代码
时间: 2024-01-14 12:04:59 浏览: 21
根据错误提示,我们需要在创建 T2 对象时传入一个 T1 类型的参数。因此,需要修改下面这行代码:
```
T2 t2 = new T2(t1);
```
这里已经正确地传入了 T1 类型的参数 t1,所以不需要再创建一个新的 T1 对象。因此,可以将下面这行代码删除:
```
T1 t1 = new T1();
```
最终的代码如下所示:
```
public class ThreadHomework {
public static void main(String[] args) {
T1 t1 = new T1();
T2 t2 = new T2(t1);
Thread th1 = new Thread(t1);
Thread th2 = new Thread(t2);
th1.start();
th2.start();
}
}
class T1 implements Runnable {
@Override
public void run() {
for (int i = 1; i <= 10; i++) {
synchronized (this) {
System.out.println("hello,world " + i);
notify();
try {
wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
synchronized (this) {
notify();
}
}
}
class T2 implements Runnable {
private T1 t1;
public T2(T1 t1) {
this.t1 = t1;
}
@Override
public void run() {
for (int i = 1; i <= 5; i++) {
synchronized (t1) {
try {
t1.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("hi " + i);
t1.notify();
}
}
}
}
```
相关推荐
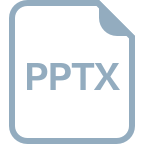
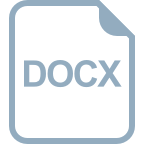
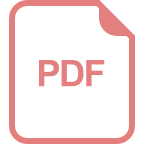















