class Test { private int data; int result = 0; public void m(){ result += 2; data += 2; System.out.print(result + " " + data); }}class ThreadExample extends Thread { private Test mv; public ThreadExample(Test mv) { this.mv = mv; } public void run(){ synchronized(mv) { mv.m(); } }}class ThreadTest{ public static void main(String args[]) { Test mv = new Test(); Thread t1 = new ThreadExample(mv); Thread t2 = new ThreadExample(mv); Thread t3 = new ThreadExample(mv); t1.start(); t2.start(); t3.start(); }}
时间: 2024-01-21 22:04:46 浏览: 93
这段代码的作用是创建一个 Test 类的实例 mv,然后创建三个线程 t1、t2、t3,并且它们共享 mv 实例。每个线程都会调用 ThreadExample 类的 run 方法,在该方法中,使用 synchronized 关键字锁定 mv 对象,然后调用 mv 对象的 m 方法,该方法会将共享变量 result 和 data 分别增加 2,并且输出它们的值。
由于使用了 synchronized 关键字对共享对象进行了同步,因此多个线程不会同时访问 mv 对象的 m 方法,从而保证了共享变量的一致性。但是,由于多个线程之间的执行顺序是不确定的,因此程序的输出结果也是不确定的。
例如,可能的输出结果是:
```
2 2
4 4
6 6
```
也可能是:
```
2 2
6 4
4 6
```
需要注意的是,如果不使用 synchronized 关键字对共享对象进行同步,那么结果就是不可预测的,可能会出现数据竞争和不正确的输出。
相关问题
class Test { private int data; int result = 0; public void m(){^ result += 2; data += 2; System.out.print(result + " " + data); } } class ThreadExample extends Thread { private Test mv; public ThreadExample(Testmv) this.mv=mv; public void run(){ synchronized(mv) mv.m(); } } } class ThreadTest{ public static void main(String args[]) { Test mv = new Test(); Thread t1 =new ThreadExample(mv); Thread t2 = new ThreadExample(mv); Thread t3 = new ThreadExample(mv) t1.start(); t2.start(); t3.start();}}的结果
这段代码存在语法错误,无法编译通过。具体来说:
1. 在 Test 类的 m 方法中,有一个多余的 ^ 符号。
2. 在 ThreadExample 类的构造方法中,Test 类的对象参数名称应该为 mv,而不是 Testmv。
3. 在 ThreadTest 类的 main 方法中,创建 ThreadExample 类的对象时,缺少了一个分号。
修复后的代码如下:
```
class Test {
private int data;
int result = 0;
public void m(){
result += 2;
data += 2;
System.out.print(result + " " + data);
}
}
class ThreadExample extends Thread {
private Test mv;
public ThreadExample(Test mv) {
this.mv = mv;
}
public void run(){
synchronized(mv) {
mv.m();
}
}
}
class ThreadTest{
public static void main(String args[]) {
Test mv = new Test();
Thread t1 = new ThreadExample(mv);
Thread t2 = new ThreadExample(mv);
Thread t3 = new ThreadExample(mv);
t1.start();
t2.start();
t3.start();
}
}
```
运行结果可能是不确定的,因为多个线程同时访问了 Test 对象的共享变量 result 和 data,而且没有对它们进行同步操作。因此,程序可能会输出不同的结果。
c#窗体应用程序实现当软件开启,定时器就在数据库扫描是否有设置的良品和不良品条码的当天ICT测试记录(ICT_test表中Barcode,Date,Result),良品和不良品条码在本地文件夹txt文件中获取。如果数据库中有良品和不良品测试记录存在,lable状态栏变绿并提示“已点检,请进行测试”,然后进行别的条码的ICT测试。(条码先扫描到c#窗体软件txt_barcode中,在通过模拟键盘定位发送到ICT测试软件条码框中,然后进行ICT测试)。如果没有,label状态栏变红,并提示“请进行测试前点检”,然后就先进行设置条码的ICT测试,判断扫描到txt_Barcode条码是否为获取到设置的良品或不良品条码,如输入的条码不符合,label状态栏变红,并提示“条码错误”,如输入的条码正确并为设置的良品条码,label状态栏变绿,并提示“请进行良品点检”,进行ICT测试,当发现某一文件夹中有良品测试报告生成,表示良品测试完成,测试完成后就提示“良品点检完成”。如扫描的是设置的不良品条码,则label状态栏变绿,并提示“请进行不良品点检”,进行ICT测试。当发现某一文件夹中有不良品测试报告生成,表示不良品测试完成。当这设置的两个条码都测试完成后,将测试记录直接更新到数据库,不需要点击上传c#代码实现.
以下是一个简单的C#窗体应用程序实现上述功能的代码:
```csharp
using System;
using System.Data.SqlClient;
using System.IO;
using System.Linq;
using System.Windows.Forms;
namespace ICTTestApplication
{
public partial class MainForm : Form
{
private Timer timer;
private string goodBarcodeFile = "good_barcodes.txt";
private string badBarcodeFile = "bad_barcodes.txt";
private string connectionString = "Data Source=SERVER_NAME;Initial Catalog=DATABASE_NAME;User ID=USERNAME;Password=PASSWORD";
public MainForm()
{
InitializeComponent();
// 设置定时器
timer = new Timer();
timer.Interval = 10000; // 10秒扫描一次
timer.Tick += new EventHandler(Timer_Tick);
timer.Enabled = true;
}
private void Timer_Tick(object sender, EventArgs e)
{
// 扫描数据库中是否有良品和不良品测试记录
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand("SELECT COUNT(*) FROM ICT_test WHERE Date = @date AND Barcode IN (SELECT Barcode FROM good_barcodes UNION SELECT Barcode FROM bad_barcodes) AND Result IS NOT NULL", connection);
command.Parameters.AddWithValue("@date", DateTime.Today);
int count = (int)command.ExecuteScalar();
if (count > 0)
{
// 有测试记录存在
statusLabel.Text = "已点检,请进行测试";
statusLabel.ForeColor = System.Drawing.Color.Green;
}
else
{
// 没有测试记录存在
statusLabel.Text = "请进行测试前点检";
statusLabel.ForeColor = System.Drawing.Color.Red;
}
}
}
private void barcodeTextBox_KeyPress(object sender, KeyPressEventArgs e)
{
if (e.KeyChar == (char)Keys.Enter)
{
string barcode = barcodeTextBox.Text.Trim();
if (File.ReadAllLines(goodBarcodeFile).Contains(barcode))
{
// 良品条码
statusLabel.Text = "请进行良品点检";
statusLabel.ForeColor = System.Drawing.Color.Green;
// 在这里进行ICT测试
// ...
// 检查是否有良品测试报告生成
if (Directory.GetFiles(@"C:\test_results\", "*_good.txt").Any())
{
// 良品测试完成
statusLabel.Text = "良品点检完成";
statusLabel.ForeColor = System.Drawing.Color.Black;
// 更新数据库中的测试记录
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand("UPDATE ICT_test SET Result = 'PASS' WHERE Date = @date AND Barcode = @barcode", connection);
command.Parameters.AddWithValue("@date", DateTime.Today);
command.Parameters.AddWithValue("@barcode", barcode);
command.ExecuteNonQuery();
}
}
}
else if (File.ReadAllLines(badBarcodeFile).Contains(barcode))
{
// 不良品条码
statusLabel.Text = "请进行不良品点检";
statusLabel.ForeColor = System.Drawing.Color.Green;
// 在这里进行ICT测试
// ...
// 检查是否有不良品测试报告生成
if (Directory.GetFiles(@"C:\test_results\", "*_bad.txt").Any())
{
// 不良品测试完成
statusLabel.Text = "不良品点检完成";
statusLabel.ForeColor = System.Drawing.Color.Black;
// 更新数据库中的测试记录
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
SqlCommand command = new SqlCommand("UPDATE ICT_test SET Result = 'FAIL' WHERE Date = @date AND Barcode = @barcode", connection);
command.Parameters.AddWithValue("@date", DateTime.Today);
command.Parameters.AddWithValue("@barcode", barcode);
command.ExecuteNonQuery();
}
}
}
else
{
// 条码错误
statusLabel.Text = "条码错误";
statusLabel.ForeColor = System.Drawing.Color.Red;
}
barcodeTextBox.Text = "";
}
}
}
}
```
需要注意的是,以上代码仅仅是一个简单的示例,实际应用中需要根据具体需求进行修改和完善。同时,为了保证代码的安全性和稳定性,建议使用参数化查询来避免 SQL 注入攻击。
阅读全文
相关推荐
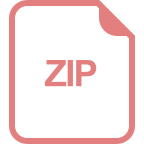
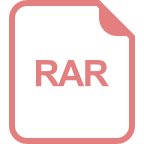
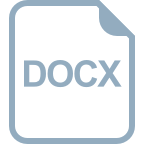
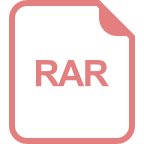
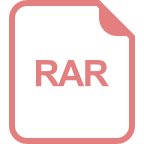
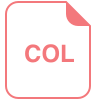
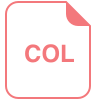
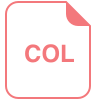
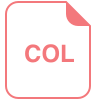
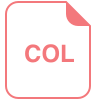
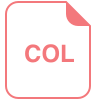
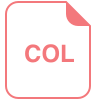
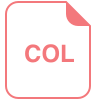


