实现一个新闻管理系统。 1: 一条新闻要求有 title;// 新闻标题 author;// 新闻作者 content;// 新闻内容 提示:1. 用1个类表示新闻信息 这个类 包含 3个属性 分别表示新闻标题 、新闻作者和新闻内容 2. 用另1个类来实现对新闻的管理 2: 系统要实现的功能有: 1. 添加新闻 :要求能添加多条新闻 2. 查询新闻 :查询全部的新闻。 3. 退出 : 3: 该系统 要求提供 返回主菜单的功能,例如: 1. 系统启动后显示主菜单: ------新闻管理系统------- 1. 添加新闻 2. 查询新闻 3. 退出 请选择 1- 3 2. 进入 子功能 显示: 请输入标题: title1 请输入作者: author1 请输入内容: content1 是否继续添加新闻,请输入 y 或者 n : n 3. 提示返回主菜单: 是否回到主菜单(输入 n 会退出
时间: 2023-11-28 11:49:17 浏览: 35
好的,我可以帮你实现这个新闻管理系统。首先,我们需要定义一个表示新闻的类,具体实现如下:
```java
public class News {
private String title; // 新闻标题
private String author; // 新闻作者
private String content; // 新闻内容
public News(String title, String author, String content) {
this.title = title;
this.author = author;
this.content = content;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public String getContent() {
return content;
}
public void setContent(String content) {
this.content = content;
}
}
```
接下来,我们需要定义一个新闻管理系统类来实现对新闻的管理,具体实现如下:
```java
import java.util.ArrayList;
import java.util.Scanner;
public class NewsManagementSystem {
private ArrayList<News> newsList; // 新闻列表
public NewsManagementSystem() {
this.newsList = new ArrayList<>();
}
public void addNews() {
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.print("请输入标题:");
String title = scanner.nextLine();
System.out.print("请输入作者:");
String author = scanner.nextLine();
System.out.print("请输入内容:");
String content = scanner.nextLine();
News news = new News(title, author, content);
newsList.add(news);
System.out.print("是否继续添加新闻,请输入 y 或者 n :");
String choice = scanner.nextLine();
if (choice.equals("n")) {
break;
}
}
}
public void queryNews() {
System.out.println("全部新闻:");
for (News news : newsList) {
System.out.println(news.getTitle() + " " + news.getAuthor() + " " + news.getContent());
}
}
public static void main(String[] args) {
NewsManagementSystem system = new NewsManagementSystem();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("------新闻管理系统-------");
System.out.println("1. 添加新闻");
System.out.println("2. 查询新闻");
System.out.println("3. 退出");
System.out.print("请选择 1-3:");
String choice = scanner.nextLine();
switch (choice) {
case "1":
system.addNews();
break;
case "2":
system.queryNews();
break;
case "3":
System.out.print("是否回到主菜单(输入 n 会退出):");
String backChoice = scanner.nextLine();
if (backChoice.equals("n")) {
System.exit(0);
}
break;
default:
System.out.println("输入有误,请重新选择!");
}
}
}
}
```
现在,你可以运行这个程序,来体验一下这个新闻管理系统。
相关推荐
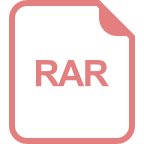
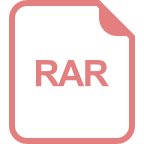
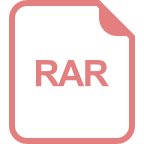














