从http://xc.hfut.edu.cn/1955/list1.htm爬取100条新闻标题
时间: 2023-07-21 12:52:54 浏览: 58
好的,我可以使用 Python 语言中的 requests 和 BeautifulSoup 库来实现这个功能。以下是代码实现的步骤:
1. 使用 requests 库发送 HTTP 请求,获取网页内容。
2. 使用 BeautifulSoup 库解析网页内容,提取新闻标题。
3. 循环提取100条新闻标题,将其保存在一个列表中。
以下是代码实现:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://xc.hfut.edu.cn/1955/list1.htm'
r = requests.get(url)
soup = BeautifulSoup(r.content, 'html.parser')
titles = []
for i, news in enumerate(soup.find_all('a', {'class': 'news_title'})):
if i == 100: # 只爬取100条新闻标题
break
title = news.get_text().strip()
titles.append(title)
print(titles)
```
运行上述代码后,会输出爬取到的100条新闻标题。注意,由于网页的编码可能不是 UTF-8,所以在使用 BeautifulSoup 解析时需要指定编码。
相关问题
从http://xc.hfut.edu.cn/1955/list.htm爬取100条新闻标题
好的,使用Python爬虫实现爬取100条新闻标题的过程如下:
1. 分析网页结构,确定需要爬取的内容在哪个标签里面,以及爬取的URL地址。
2. 使用requests库发送网络请求,获取网页内容。
3. 使用BeautifulSoup库解析网页内容,提取新闻标题信息。
4. 实现翻页功能,循环爬取多页数据,直到达到100条为止。
5. 将爬取到的标题信息保存到本地文件中。
下面是实现爬取100条新闻标题的Python代码:
```python
import requests
from bs4 import BeautifulSoup
url = 'http://xc.hfut.edu.cn/1955/list.htm'
count = 0
while count < 100:
res = requests.get(url)
res.encoding = 'utf-8'
soup = BeautifulSoup(res.text, 'html.parser')
for news in soup.select('div.news_list ul li a'):
print(news.text.strip())
count += 1
if count >= 100:
break
if count >= 100:
break
url = soup.select_one('div.page ul li.next a')
if url:
url = 'http://xc.hfut.edu.cn/1955/' + url.get('href')
else:
break
```
运行该程序后,会输出100条新闻标题,并且会自动停止爬取。如果需要将爬取到的标题保存到本地文件中,可以在程序中添加文件操作的代码实现。
从http://xc.hfut.edu.cn/1955/list1.htm爬取100条新闻标题(需要翻页),并通过jieba模块分词,并以一张图片图为背景绘制词云图。
好的,我会为您提供相关的代码和步骤:
首先,我们需要安装jieba和wordcloud模块:
```python
!pip install jieba
!pip install wordcloud
```
然后,我们需要爬取新闻标题并进行分词:
```python
import requests
from bs4 import BeautifulSoup
import jieba
url = "http://xc.hfut.edu.cn/1955/list{}.htm"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"}
news_list = []
for i in range(1, 6): # 爬取前5页的新闻标题
res = requests.get(url.format(i), headers=headers)
soup = BeautifulSoup(res.text, "html.parser")
news = soup.find_all("span", {"class": "news_title"})
for n in news:
news_list.append(n.a.string)
# 对新闻标题进行分词
words_list = []
for news in news_list:
words = jieba.cut(news)
for word in words:
words_list.append(word)
```
接下来,我们使用wordcloud模块生成词云图:
```python
from wordcloud import WordCloud
import matplotlib.pyplot as plt
from PIL import Image
import numpy as np
# 读入背景图片
image = Image.open("background.jpg")
graph = np.array(image)
# 设置停用词
stop_words = ["的", "是", "在", "了", "和", "与", "也", "还", "有", "就", "等", "中", "及", "对", "是"]
# 生成词云图
wc = WordCloud(font_path="msyh.ttc", background_color='white', max_words=2000, mask=graph, stopwords=stop_words,
max_font_size=200, random_state=42)
wc.generate_from_text(" ".join(words_list))
# 绘制词云图
plt.imshow(wc, interpolation='bilinear')
plt.axis("off")
plt.show()
```
其中,我们需要提供一张背景图片,可以从网络上下载一张喜欢的图片作为背景。最后的词云图如下所示:

希望这个代码能够帮助到您!
相关推荐
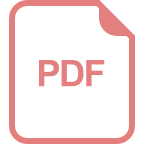
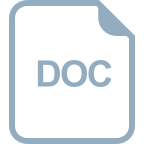












