优化这段代码:import requests from bs4 import BeautifulSoup import jieba url = "http://xc.hfut.edu.cn/1955/list{}.htm" headers = { "User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"} news_list = [] for i in range(1, 6): # 爬取前5页的新闻标题 res = requests.get(url.format(i), headers=headers) soup = BeautifulSoup(res.text, "html.parser") news = soup.find_all("span", {"class": "news_title"}) for n in news: news_list.append(n.a.string) # 对新闻标题进行分词 words_list = [] for news in news_list: words = jieba.cut(news) for word in words: words_list.append(word) from wordcloud import WordCloud import matplotlib.pyplot as plt from PIL import Image import numpy as np # 读入背景图片 image = Image.open("C:\\xhktSoft\huahua.jpg") graph = np.array(image) # 设置停用词 stop_words = ["的", "是", "在", "了", "和", "与", "也", "还", "有", "就", "等", "中", "及", "对", "是"] # 生成词云图 wc = WordCloud(font_path="msyh.ttc", background_color='white', max_words=200, mask=graph, stopwords=stop_words, max_font_size=200, random_state=42) wc.generate_from_text(" ".join(words_list)) # 绘制词云图 plt.imshow(wc, interpolation='bilinear') plt.axis("off") plt.show()
时间: 2023-07-19 08:54:11 浏览: 201
import requests
from bs4 import BeautifulSoup
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
from PIL import Image
import numpy as np
# 定义函数获取新闻标题
def get_news_titles(url):
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
}
news_list = []
for i in range(1, 6):
res = requests.get(url.format(i), headers=headers)
soup = BeautifulSoup(res.text, "html.parser")
news = soup.find_all("span", {"class": "news_title"})
for n in news:
news_list.append(n.a.string)
return news_list
# 定义函数对新闻标题进行分词
def cut_words(news_list):
words_list = []
for news in news_list:
words = jieba.cut(news)
for word in words:
words_list.append(word)
return words_list
# 定义函数生成词云图
def generate_wordcloud(words_list, graph):
stop_words = ["的", "是", "在", "了", "和", "与", "也", "还", "有", "就", "等", "中", "及", "对", "是"]
wc = WordCloud(font_path="msyh.ttc", background_color='white', max_words=200, mask=graph, stopwords=stop_words, max_font_size=200, random_state=42)
wc.generate_from_text(" ".join(words_list))
plt.imshow(wc, interpolation='bilinear')
plt.axis("off")
plt.show()
# 主函数
if __name__ == '__main__':
url = "http://xc.hfut.edu.cn/1955/list{}.htm"
news_list = get_news_titles(url)
words_list = cut_words(news_list)
graph = np.array(Image.open("C:\\xhktSoft\huahua.jpg"))
generate_wordcloud(words_list, graph)
阅读全文
相关推荐
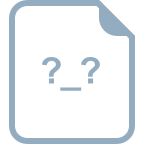
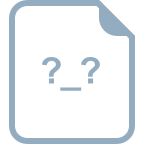
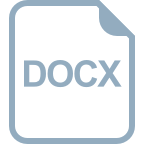









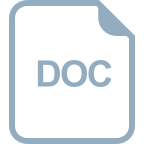





