本题要求用C语言实现带头结点的循环单链表的创建和单链表的区间删除。L是一个带头结点的循环单链表,函数ListCreate_CL用于创建一个循环单链表,函数ListDelete_CL用于删除取值大于min小于max的链表元素
时间: 2024-10-12 17:07:11 浏览: 6
在C语言中,我们可以使用结构体表示链表节点,同时定义两个函数`ListCreate_CL()` 和 `ListDelete_CL()` 来分别实现循环单链表的创建和区间删除操作。首先,我们需要定义链表节点结构:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建一个带头结点的循环链表
Node* ListCreate_CL() {
// 初始化头结点
Node* head = (Node*)malloc(sizeof(Node));
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = 0; // 将头节点设为空或0
head->next = head; // 设置头节点指向自身形成循环
// 你可以添加更多节点到链表...
// 这里仅作为示例,实际实现会根据需要追加新节点
return head;
}
// 区间删除链表元素(min和max已知)
void ListDelete_CL(Node* head, int min, int max) {
Node* current = head;
// 遍历链表直到找到第一个大于min的节点
while (current->data < min && current != head) {
current = current->next;
}
if (current == head) { // 如果所有元素都小于min,则无需删除
return;
}
Node* prev = current->prev; // 记录当前节点的前一个节点
Node* temp = current;
// 删除满足条件的节点
while (temp->data > min && temp->data < max && temp != head) {
prev->next = temp->next;
temp->next->prev = prev;
temp = temp->next;
}
// 如果删除到了头节点,则更新头节点的下一个指针
if (temp == head) {
head->next = current;
} else {
temp->prev->next = temp->next;
}
}
```
相关推荐
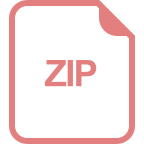
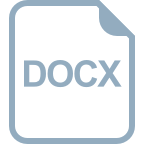
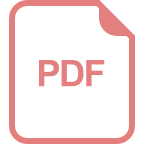














