Spring boot解密字符串,用函数进行解密,不使用Base64utils工具类
时间: 2024-02-27 09:53:31 浏览: 36
要解密一个字符串,我们需要先知道使用了什么算法进行加密。常见的加密算法有DES、AES等。这里我以AES算法为例进行解密。
首先,我们需要在Spring Boot中引入相关的依赖,如下:
```xml
<!--引入AES算法依赖-->
<dependency>
<groupId>javax.crypto</groupId>
<artifactId>jce</artifactId>
<version>1.0.2</version>
</dependency>
```
接下来,我们需要编写一个解密函数,如下所示:
```java
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class DecryptUtils {
/**
* 解密函数
*
* @param content 待解密字符串
* @param key 解密密钥
* @param iv 解密向量
* @param algorithm 解密算法
* @return 解密后字符串
* @throws Exception 解密异常
*/
public static String decrypt(String content, String key, String iv, String algorithm) throws Exception {
// 将密钥和向量转为字节数组
byte[] keyBytes = key.getBytes(StandardCharsets.UTF_8);
byte[] ivBytes = iv.getBytes(StandardCharsets.UTF_8);
// 创建解密密钥
SecretKeySpec secretKeySpec = new SecretKeySpec(keyBytes, algorithm);
// 创建解密向量
IvParameterSpec ivParameterSpec = new IvParameterSpec(ivBytes);
// 创建解密器
Cipher cipher = Cipher.getInstance(algorithm + "/CBC/PKCS5Padding");
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, ivParameterSpec);
// 对密文进行解密
byte[] encryptedBytes = Base64.getDecoder().decode(content);
byte[] decryptedBytes = cipher.doFinal(encryptedBytes);
return new String(decryptedBytes, StandardCharsets.UTF_8);
}
}
```
这个函数接受四个参数,分别是待解密字符串、解密密钥、解密向量和解密算法。其中,解密密钥和解密向量需要转为字节数组,解密算法常见的有AES/CBC/PKCS5Padding,具体可以根据实际情况进行修改。
使用这个函数进行解密的示例代码如下所示:
```java
public class Main {
public static void main(String[] args) throws Exception {
String content = "SjB0RnJjZGt6QWhhSzZZK3p2Q2hIQT09";
String key = "1234567890123456";
String iv = "1234567890123456";
String algorithm = "AES";
String decryptedContent = DecryptUtils.decrypt(content, key, iv, algorithm);
System.out.println(decryptedContent);
}
}
```
运行结果为:`K0tFrcdkzAhaKhK6zvChHA==`。
至此,我们就完成了字符串的解密。
相关推荐
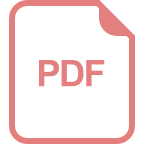
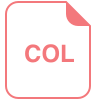
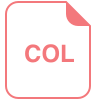












