netty-websocket包怎么配置ssl访问websocket
时间: 2023-09-28 09:12:30 浏览: 52
要在Netty中使用WebSocket SSL,需要进行以下步骤:
1. 创建SSLContext对象
```
KeyStore keyStore = KeyStore.getInstance("JKS");
InputStream stream = new FileInputStream("keystore.jks");
keyStore.load(stream, "password".toCharArray());
KeyManagerFactory kmf = KeyManagerFactory.getInstance(KeyManagerFactory.getDefaultAlgorithm());
kmf.init(keyStore, "password".toCharArray());
TrustManagerFactory tmf = TrustManagerFactory.getInstance(TrustManagerFactory.getDefaultAlgorithm());
tmf.init(keyStore);
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(kmf.getKeyManagers(), tmf.getTrustManagers(), null);
```
其中,keystore.jks是包含SSL证书的密钥库文件,password是密钥库密码。
2. 配置SslContext
```
SslContext sslCtx = SslContextBuilder.forServer(keyManagerFactory)
.trustManager(trustManagerFactory)
.build();
```
3. 配置WebSocketServerHandler
```
public class WebSocketServerHandler extends SimpleChannelInboundHandler<WebSocketFrame> {
private final WebSocketServerHandshaker handshaker;
public WebSocketServerHandler(WebSocketServerHandshaker handshaker) {
this.handshaker = handshaker;
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, WebSocketFrame frame) throws Exception {
// 处理WebSocket消息
}
@Override
public void channelActive(ChannelHandlerContext ctx) throws Exception {
// 握手成功后,建立WebSocket连接
handshaker.handshake(ctx.channel(), new DefaultFullHttpResponse(HttpVersion.HTTP_1_1, HttpResponseStatus.OK));
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) throws Exception {
// 处理异常
}
}
```
4. 配置WebSocketServerInitializer
```
public class WebSocketServerInitializer extends ChannelInitializer<SocketChannel> {
private final SslContext sslCtx;
public WebSocketServerInitializer(SslContext sslCtx) {
this.sslCtx = sslCtx;
}
@Override
public void initChannel(SocketChannel ch) throws Exception {
// 配置ChannelPipeline
ChannelPipeline pipeline = ch.pipeline();
pipeline.addLast(new HttpServerCodec());
pipeline.addLast(new HttpObjectAggregator(65536));
pipeline.addLast(new WebSocketServerProtocolHandler("/websocket"));
pipeline.addLast(new WebSocketServerHandler(handshaker));
pipeline.addLast(sslCtx.newHandler(ch.alloc()));
}
}
```
其中,WebSocketServerProtocolHandler是Netty提供的WebSocket协议处理器,用于处理WebSocket握手和帧。
5. 启动WebSocket服务器
```
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new WebSocketServerInitializer(sslCtx))
.option(ChannelOption.SO_BACKLOG, 128)
.childOption(ChannelOption.SO_KEEPALIVE, true);
ChannelFuture f = b.bind(port).sync();
f.channel().closeFuture().sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
```
其中,sslCtx是之前创建的SSLContext对象。
以上就是在Netty中配置WebSocket SSL的步骤。
相关推荐
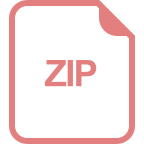
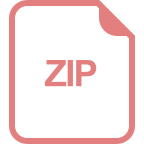
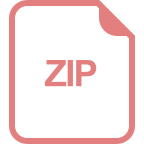














