用java对AOP的实现代码示例
时间: 2024-03-20 19:40:59 浏览: 60
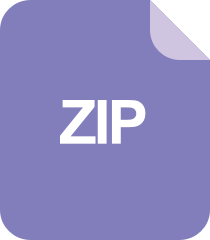
实现AOP的一个例子
好的,以下是一个简单的使用Java实现AOP的示例代码:
首先,定义一个切面类,实现需要在目标方法执行前和执行后进行的逻辑:
```java
public class LoggingAspect {
public void beforeAdvice(JoinPoint joinPoint) {
System.out.println("Before executing " + joinPoint.getSignature().getName() + " method.");
}
public void afterAdvice(JoinPoint joinPoint) {
System.out.println("After executing " + joinPoint.getSignature().getName() + " method.");
}
}
```
然后,在需要进行AOP的目标类中,使用注解标注需要进行切面的方法:
```java
public class MyService {
@Loggable
public void doSomething() {
System.out.println("Doing something.");
}
}
```
接着,定义一个切入点表达式,指定需要拦截的方法:
```java
@Pointcut("@annotation(com.example.Loggable)")
public void loggableMethod() {}
```
最后,在Spring配置文件中,定义切面和目标类的bean,并将切面和目标类的bean配置为代理对象:
```xml
<bean id="loggingAspect" class="com.example.LoggingAspect"/>
<bean id="myService" class="com.example.MyService"/>
<aop:config>
<aop:aspect ref="loggingAspect">
<aop:pointcut expression="loggableMethod()"/>
<aop:before method="beforeAdvice" pointcut-ref="loggableMethod"/>
<aop:after method="afterAdvice" pointcut-ref="loggableMethod"/>
</aop:aspect>
</aop:config>
<bean id="myServiceProxy" class="org.springframework.aop.framework.ProxyFactoryBean">
<property name="target" ref="myService"/>
<property name="interceptorNames">
<list>
<value>loggingAspect</value>
</list>
</property>
</bean>
```
这样,当调用MyService中使用@Loggable注解标注的方法时,LoggingAspect中的beforeAdvice和afterAdvice方法会分别在目标方法执行前和执行后被调用。
阅读全文
相关推荐
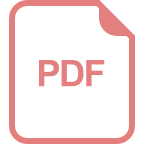
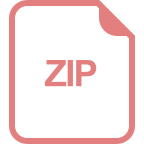
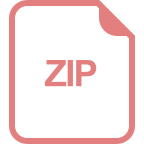
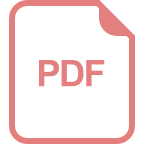
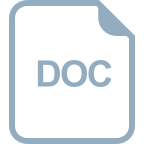
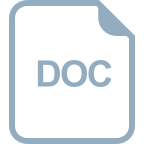
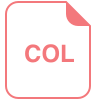




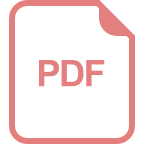
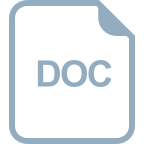
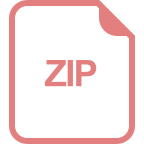
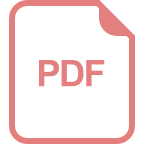
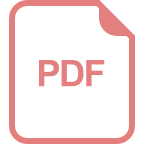