package org.example.application.custom.controller; import lombok.extern.slf4j.Slf4j; import org.example.application.common.ResponseDTO; import org.example.application.common.utils.jwt.JwtIgnore; import org.example.application.custom.entity.Free; import org.example.application.custom.form.FreeForm; import org.example.application.custom.service.FreeService; import org.springframework.web.bind.annotation.PathVariable; import org.springframework.web.bind.annotation.RequestBody; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; import java.util.List; @RestController @RequestMapping(value = "/api/free") @Slf4j public class FreeController { private final FreeService freeService; public FreeController(FreeService freeService) { this.freeService = freeService; } @RequestMapping("/list/mine") public ResponseDTO queryMine(@RequestBody FreeForm form) { Iterable<Free> list = freeService.queryMineList(form); return ResponseDTO.returnSuccess(list); } @RequestMapping("/list/check") public ResponseDTO queryCheck(@RequestBody FreeForm form) { Iterable<Free> list = freeService.queryCheckList(form); return ResponseDTO.returnSuccess(list); } @RequestMapping("/save") public ResponseDTO save(@RequestBody FreeForm form) { freeService.save(form); return ResponseDTO.returnSuccess(); } @RequestMapping("/get/{id}") public ResponseDTO getById(@PathVariable String id) { Free entity = freeService.getById(id); return ResponseDTO.returnSuccess(entity); } @RequestMapping("/delete/{id}") public ResponseDTO deleteById(@PathVariable String id) { freeService.deleteById(id); return ResponseDTO.returnSuccess(); } @RequestMapping("/check/{id}/{status}") public ResponseDTO check(@PathVariable String id, @PathVariable Integer status) { freeService.check(id, status); return ResponseDTO.returnSuccess(); } }
时间: 2024-04-02 18:35:11 浏览: 160
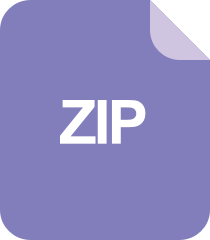
slf4j最新jar包下载和jar包

这段代码是一个 Spring Boot 的 Controller 类,位于 org.example.application.custom.controller 包下。它引入了 lombok、ResponseDTO、JwtIgnore、Free、FreeForm 和 FreeService 类。该类定义了一个 RestController,映射了 /api/free 路径,提供了 queryMine()、queryCheck()、save()、getById()、deleteById() 和 check() 等接口。其中,queryMine() 和 queryCheck() 方法用于查询自己和待审核的 Free 记录,save() 方法用于保存 Free 记录,getById() 方法用于根据 id 查询 Free 记录,deleteById() 方法用于根据 id 删除 Free 记录,check() 方法用于审核 Free 记录。该类使用了 @Slf4j 注解,表示使用了 lombok 提供的日志功能。
阅读全文
相关推荐
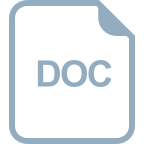















