用C语言写一个watchdog
时间: 2023-08-23 18:03:31 浏览: 94
要编写一个 watchdog 程序,你需要使用系统调用来设置定时器并检查进程的状态。以下是一个简单的 C 代码示例,可以在 Linux 系统上实现 watchdog:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <signal.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <string.h>
#define WATCHDOG_TIMEOUT 30 // 监视器超时时间为30秒
void handle_signal(int signal) {
printf("Watchdog: Process is running\n");
}
int main(int argc, char *argv[]) {
pid_t pid;
int fd;
char buf[256];
if(argc < 2) {
fprintf(stderr, "Usage: %s <program to watch>\n", argv[0]);
return EXIT_FAILURE;
}
pid = fork();
if (pid < 0) {
perror("fork");
return EXIT_FAILURE;
} else if (pid == 0) {
/* 子进程 */
execvp(argv[1], &argv[1]);
perror("execvp");
return EXIT_FAILURE;
}
/* 父进程 */
fd = open("/dev/watchdog", O_WRONLY);
if (fd == -1) {
perror("open");
return EXIT_FAILURE;
}
printf("Watchdog: Starting %s with pid %d\n", argv[1], pid);
while (1) {
signal(SIGUSR1, handle_signal); // 捕捉 SIGUSR1 信号
write(fd, "a", 1); // 向 watchdog 设备发送信号
sleep(WATCHDOG_TIMEOUT); // 等待 watchdog 超时
if (kill(pid, 0) == -1) { // 检查进程是否存在
printf("Watchdog: Process %d is not running, exiting\n", pid);
break;
}
}
close(fd);
return EXIT_SUCCESS;
}
```
在这个示例中,watchdog 程序使用 fork() 创建一个子进程来运行需要监视的程序,然后使用 open() 函数打开 watchdog 设备文件 "/dev/watchdog"。程序进入一个无限循环,使用 write() 函数向 watchdog 设备发送信号并等待 watchdog 超时。如果进程被杀死或崩溃,那么在下一次检查时 kill() 函数将返回 -1,程序将退出。
你可以将这个程序编译成可执行文件并在终端上运行:
```
$ gcc -o watchdog watchdog.c
$ ./watchdog my_program
```
这将监视 "my_program" 进程并在进程崩溃或被杀死时重新启动它。
阅读全文
相关推荐
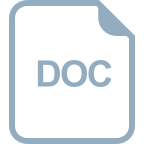
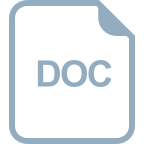
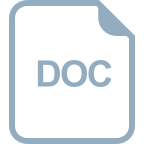






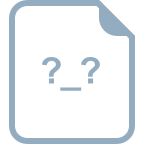
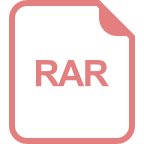
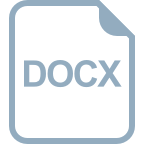
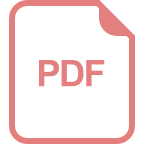
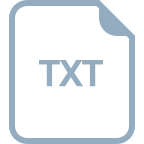
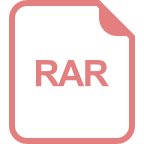
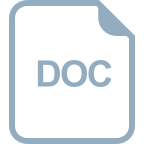


