c语言字符串的定义需要加大括号吗
时间: 2023-08-21 14:04:16 浏览: 221
在 C 语言中,定义字符串时不需要加大括号。因为字符串是一个字符数组,可以使用赋值语句或字符串常量来初始化,不需要使用大括号。
例如,以下定义字符串的代码是合法的:
```c
char str[] = "hello"; // 直接定义字符串常量
char str2[6] = {'h', 'e', 'l', 'l', 'o', '\0'}; // 使用字符数组赋值初始化
char *str3 = "hello"; // 使用指针定义字符串
```
可以看到,这些代码中都没有使用大括号来定义字符串。
需要注意的是,当使用字符数组赋值初始化字符串时,需要显式地在最后一个字符后加上空字符 `'\0'`,否则该字符串可能不会被正确地结束,导致程序出错。
相关问题
c语言查找字符串括号中间的字符串
可以利用栈来实现查找字符串括号中间的字符串。具体步骤如下:
1. 定义一个栈,用于存储左括号的下标。
2. 遍历字符串,遇到左括号时,将其下标入栈。
3. 遇到右括号时,弹出栈顶元素,该元素对应的下标即为左括号的下标。
4. 截取左括号和右括号之间的子串,即为括号中间的字符串。
以下是示例代码:
```c
#include <stdio.h>
#include <string.h>
#define STACK_SIZE 100
int stack[STACK_SIZE];
int top = -1;
void push(int x)
{
if (top == STACK_SIZE - 1) {
printf("Stack overflow!\n");
return;
}
stack[++top] = x;
}
int pop()
{
if (top == -1) {
printf("Stack underflow!\n");
return -1;
}
return stack[top--];
}
int main()
{
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] == '(') {
push(i);
} else if (str[i] == ')') {
int left = pop();
if (left != -1) {
printf("Substring between parentheses: %.*s\n", i - left - 1, str + left + 1);
}
}
}
return 0;
}
```
输入示例:
```
Enter a string: hello(world)how(are(you))
```
输出示例:
```
Substring between parentheses: world
Substring between parentheses: are
Substring between parentheses: you
```
c语言字符串数组初始化并赋值
可以使用以下两种方式进行 C 语言字符串数组初始化并赋值:
1. 使用花括号 {} 进行初始化
```c
char str_arr[][20] = {"Hello", "world", "!"};
```
上述代码中,str_arr 是一个二维字符数组,第一维无需指定大小,第二维指定为 20,表示每个字符串的长度不超过 19 个字符。使用花括号初始化时,可以不指定第一维的大小,编译器会自动计算出数组的大小。
2. 逐个赋值
```c
char str_arr[3][20];
strcpy(str_arr[0], "Hello");
strcpy(str_arr[1], "world");
strcpy(str_arr[2], "!");
```
上述代码中,先定义了一个二维字符数组 str_arr,然后使用 strcpy 函数逐个赋值。需要注意的是,第二维的大小必须大于等于最长的字符串长度加一,即 20 大于等于 "world" 的长度 5,加一得到 6。
阅读全文
相关推荐
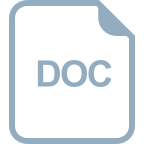
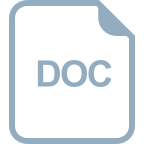
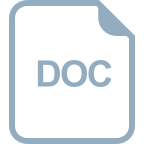
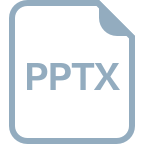
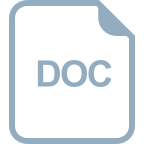

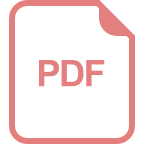
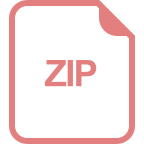
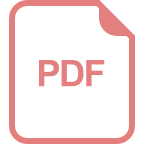
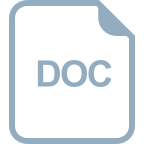
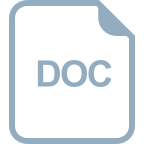
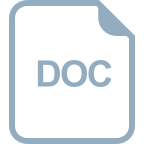





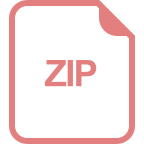