c语言用栈写一个括号匹配
时间: 2023-09-11 22:06:29 浏览: 107
以下是一个使用栈来判断括号匹配的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define STACK_SIZE 100
//定义栈结构体
typedef struct {
char data[STACK_SIZE];
int top;
} Stack;
//初始化栈
void initStack(Stack *s) {
s->top = -1;
}
//判断栈是否为空
int isEmpty(Stack *s) {
return s->top == -1;
}
//判断栈是否已满
int isFull(Stack *s) {
return s->top == STACK_SIZE - 1;
}
//入栈
void push(Stack *s, char c) {
if (isFull(s)) {
printf("Stack is full.\n");
exit(1);
}
s->data[++s->top] = c;
}
//出栈
char pop(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty.\n");
exit(1);
}
return s->data[s->top--];
}
//获取栈顶元素
char peek(Stack *s) {
if (isEmpty(s)) {
printf("Stack is empty.\n");
exit(1);
}
return s->data[s->top];
}
//判断括号是否匹配
int isMatch(char left, char right) {
if (left == '(' && right == ')')
return 1;
else if (left == '[' && right == ']')
return 1;
else if (left == '{' && right == '}')
return 1;
else
return 0;
}
//判断整个字符串中的括号是否匹配
int isBalanced(char *s) {
Stack stack;
initStack(&stack);
int len = strlen(s);
for (int i = 0; i < len; i++) {
char c = s[i];
if (c == '(' || c == '[' || c == '{') {
push(&stack, c);
} else if (c == ')' || c == ']' || c == '}') {
if (isEmpty(&stack))
return 0;
char left = pop(&stack);
if (!isMatch(left, c))
return 0;
}
}
return isEmpty(&stack);
}
int main() {
char s[100];
printf("Enter a string: ");
scanf("%s", s);
if (isBalanced(s)) {
printf("The string is balanced.\n");
} else {
printf("The string is not balanced.\n");
}
return 0;
}
```
程序先定义了一个栈结构体,并实现了相关的栈操作函数。其中,isMatch函数用于判断左右括号是否匹配,isBalanced函数用于判断整个字符串中的括号是否匹配。
在main函数中,程序首先读入一个字符串,然后调用isBalanced函数判断该字符串中的括号是否匹配,并输出相应的结果。
阅读全文
相关推荐








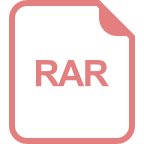
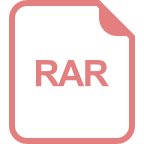







