用c++写一个括号匹配的代码
时间: 2024-05-09 08:18:37 浏览: 70
以下是用C语言实现括号匹配的代码:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100 //定义一个栈的最大容量
//定义一个栈结构体
typedef struct {
char data[MAX_SIZE];
int top; //栈顶指针
} Stack;
//初始化栈
void initStack(Stack *s) {
s->top = -1; //栈顶指针初始化为-1,表示栈为空
}
//入栈
void push(Stack *s, char c) {
if (s->top == MAX_SIZE - 1) { //栈满了
printf("Stack overflow!\n");
exit(1);
}
s->data[++s->top] = c; //栈顶指针先加1,再将元素入栈
}
//出栈
char pop(Stack *s) {
if (s->top == -1) { //栈空了
printf("Stack underflow!\n");
exit(1);
}
return s->data[s->top--]; //先取出栈顶元素,再将栈顶指针减1
}
//判断栈是否为空
int isEmpty(Stack *s) {
return s->top == -1;
}
//括号匹配函数,返回1表示匹配成功,返回0表示匹配失败
int match(char *str) {
Stack s;
initStack(&s); //初始化栈
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] == '(' || str[i] == '[' || str[i] == '{') {
push(&s, str[i]); //左括号入栈
} else if (str[i] == ')' || str[i] == ']' || str[i] == '}') {
if (isEmpty(&s)) { //栈为空,右括号无法匹配
return 0;
}
char c = pop(&s); //取出栈顶元素
if ((str[i] == ')' && c != '(') || (str[i] == ']' && c != '[') || (str[i] == '}' && c != '{')) {
//右括号与栈顶元素不匹配
return 0;
}
}
}
return isEmpty(&s); //栈为空,匹配成功,否则匹配失败
}
int main() {
char str[MAX_SIZE];
printf("请输入要判断的字符串:");
scanf("%s", str);
if (match(str)) {
printf("%s是合法的表达式\n", str);
} else {
printf("%s是不合法的表达式\n", str);
}
return 0;
}
```
使用方法:输入一个要判断的字符串,程序会输出该字符串是否是合法的表达式。例如,输入字符串"({1+2*[3+4]})",程序会输出"({1+2*[3+4]})是合法的表达式"。
阅读全文
相关推荐
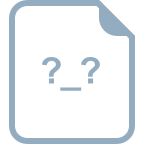


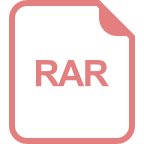
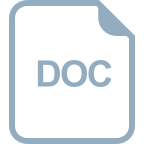









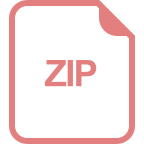