写一段安卓7.0的ble蓝牙的读写代码
时间: 2023-04-06 07:02:51 浏览: 155
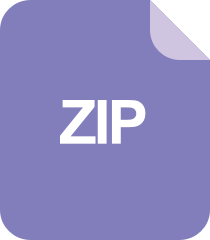
Android BLE实现对蓝牙的读写
以下是一段安卓7.0的BLE蓝牙读写代码,供参考:
```java
// 初始化蓝牙适配器
BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
BluetoothAdapter bluetoothAdapter = bluetoothManager.getAdapter();
// 获取远程设备
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(address);
// 连接远程设备
BluetoothGatt gatt = device.connectGatt(this, false, new BluetoothGattCallback() {
// 连接状态改变回调
@Override
public void onConnectionStateChange(BluetoothGatt gatt, int status, int newState) {
if (newState == BluetoothProfile.STATE_CONNECTED) {
// 连接成功,开始发现服务
gatt.discoverServices();
} else if (newState == BluetoothProfile.STATE_DISCONNECTED) {
// 连接断开,释放资源
gatt.close();
}
}
// 发现服务回调
@Override
public void onServicesDiscovered(BluetoothGatt gatt, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 获取服务
BluetoothGattService service = gatt.getService(serviceUuid);
// 获取特征值
BluetoothGattCharacteristic characteristic = service.getCharacteristic(characteristicUuid);
// 读取特征值
gatt.readCharacteristic(characteristic);
// 写入特征值
byte[] data = new byte[]{0x01, 0x02, 0x03};
characteristic.setValue(data);
gatt.writeCharacteristic(characteristic);
}
}
// 读取特征值回调
@Override
public void onCharacteristicRead(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 读取成功,获取数据
byte[] data = characteristic.getValue();
}
}
// 写入特征值回调
@Override
public void onCharacteristicWrite(BluetoothGatt gatt, BluetoothGattCharacteristic characteristic, int status) {
if (status == BluetoothGatt.GATT_SUCCESS) {
// 写入成功
}
}
});
```
阅读全文
相关推荐
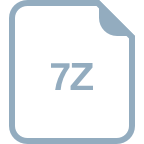
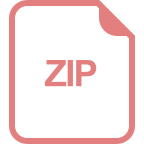
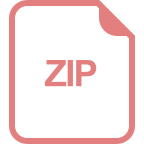
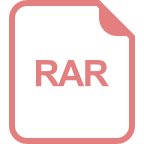

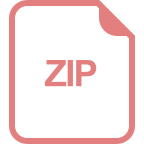
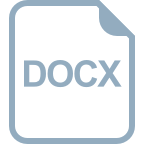
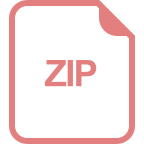
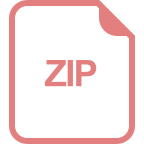
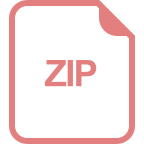
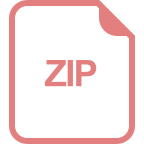
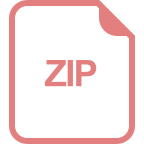