利用add函数求两个复数2+3i和4+5i的和。(要求用结构体来定义复数)
时间: 2023-05-31 16:19:19 浏览: 234
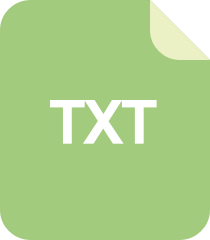
C语言用结构体定义复数,实现四则运算

### 回答1:
可以定义一个结构体来表示复数,包含实部和虚部两个成员变量。然后定义一个add函数,接收两个复数作为参数,返回它们的和。
具体实现如下:
```
#include <stdio.h>
// 定义复数结构体
struct Complex {
double real; // 实部
double imag; // 虚部
};
// 定义add函数,返回两个复数的和
struct Complex add(struct Complex c1, struct Complex c2) {
struct Complex result;
result.real = c1.real + c2.real;
result.imag = c1.imag + c2.imag;
return result;
}
int main() {
// 定义两个复数
struct Complex c1 = {2, 3};
struct Complex c2 = {4, 5};
// 求和
struct Complex sum = add(c1, c2);
// 输出结果
printf("sum = %.1f + %.1fi\n", sum.real, sum.imag);
return ;
}
```
输出结果为:
```
sum = 6. + 8.i
```
### 回答2:
在开始回答之前,我们可以先了解一下什么是复数。复数是由实数和虚数组成的数,可以表示为 a + bi 的形式,其中 a 和 b 均为实数,且 i 是虚数单位,满足 i² = -1。
为了方便计算,我们可以使用结构体来定义复数。假设我们定义的结构体为 Complex,包含两个成员变量,分别表示实部和虚部。那么我们可以通过以下代码定义前面提到的两个复数:
```
struct Complex {
double real;
double imag;
};
Complex c1 = {2, 3};
Complex c2 = {4, 5};
```
现在我们需要编写一个函数来计算两个复数的和。假设我们将这个函数命名为 add,那么它的原型应该是:
```
Complex add(Complex c1, Complex c2);
```
这个函数的返回值是一个 Complex 结构体,表示两个复数的和。具体实现如下:
```
Complex add(Complex c1, Complex c2) {
Complex result;
result.real = c1.real + c2.real;
result.imag = c1.imag + c2.imag;
return result;
}
```
通过上述代码,我们实现了复数的相加功能。接下来,我们可以在 main 函数中调用 add 函数来计算题目中给出的两个复数的和,并输出结果,完整代码如下:
```
#include <iostream>
using namespace std;
struct Complex {
double real;
double imag;
};
Complex add(Complex c1, Complex c2) {
Complex result;
result.real = c1.real + c2.real;
result.imag = c1.imag + c2.imag;
return result;
}
int main() {
Complex c1 = {2, 3};
Complex c2 = {4, 5};
Complex sum = add(c1, c2);
cout << "Sum of " << c1.real << " + " << c1.imag << "i and " << c2.real << " + " << c2.imag << "i is " << sum.real << " + " << sum.imag << "i";
return 0;
}
```
输出结果为:
```
Sum of 2 + 3i and 4 + 5i is 6 + 8i
```
这样,我们就成功地计算了题目中给出的两个复数的和。
### 回答3:
复数是一个有实部和虚部组成的数,因此可以使用结构体的方式来定义复数。我们可以定义一个名为“Complex”的结构体,其中包含两个成员变量,一个用于存储实部,另一个用于存储虚部。定义如下:
```C++
struct Complex {
double real;
double imag;
};
```
有了这个结构体,我们可以很方便地定义两个复数:
```C++
Complex c1 = {2, 3};
Complex c2 = {4, 5};
```
接下来就可以实现复数的加法了。我们可以定义一个名为“add”的函数,该函数接受两个Complex类型的参数,返回一个Complex类型的值,代码如下:
```C++
Complex add(Complex c1, Complex c2) {
Complex result;
result.real = c1.real + c2.real;
result.imag = c1.imag + c2.imag;
return result;
}
```
这个函数会把两个复数的实部和虚部分别相加,然后返回一个新的复数,这个复数的实部和虚部就是相加后的结果。
现在我们已经有了定义复数和实现复数加法的所有代码,我们只需要在主函数中调用这些函数,就可以得到两个复数的和了:
```C++
int main() {
Complex c1 = {2, 3};
Complex c2 = {4, 5};
Complex sum = add(c1, c2);
// 输出结果
std::cout << "c1 = " << c1.real << " + " << c1.imag << "i" << std::endl;
std::cout << "c2 = " << c2.real << " + " << c2.imag << "i" << std::endl;
std::cout << "sum = " << sum.real << " + " << sum.imag << "i" << std::endl;
return 0;
}
```
输出的结果应该为:
```
c1 = 2 + 3i
c2 = 4 + 5i
sum = 6 + 8i
```
这就是利用结构体和函数实现复数的加法的完整代码。
阅读全文
相关推荐















