2、定义复数类,成员如下:1) x 实部 (私有)2) y 虚部 (私有)3) 构造函数,包括有参构造函数和默认构造函数4) 析构函数5) 复制构造函数6) show函数:用于输出复数,形式为x+yi7) 并且定义友员函数add,sub,mul用于实现复数的加减乘。在主函数中,选择相应的符号进行运算。
时间: 2023-06-10 12:02:37 浏览: 110
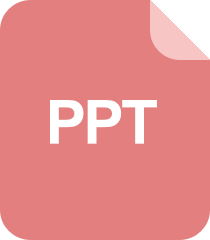
小复习—例复数构造函数重载-C++ppt-类与对象
好的,我明白了你的要求。以下是定义复数类的代码:
```
#include <iostream>
using namespace std;
class Complex {
private:
double x; // 实部
double y; // 虚部
public:
Complex() { // 默认构造函数
x = 0;
y = 0;
}
Complex(double a, double b) { // 有参构造函数
x = a;
y = b;
}
~Complex() { // 析构函数
cout << "Complex object destroyed" << endl;
}
Complex(const Complex& c) { // 复制构造函数
x = c.x;
y = c.y;
}
void show() const { // 输出复数
cout << x << " + " << y << "i" << endl;
}
friend Complex add(const Complex& c1, const Complex& c2); // 加法友元函数
friend Complex sub(const Complex& c1, const Complex& c2); // 减法友元函数
friend Complex mul(const Complex& c1, const Complex& c2); // 乘法友元函数
};
Complex add(const Complex& c1, const Complex& c2) { // 加法函数实现
return Complex(c1.x + c2.x, c1.y + c2.y);
}
Complex sub(const Complex& c1, const Complex& c2) { // 减法函数实现
return Complex(c1.x - c2.x, c1.y - c2.y);
}
Complex mul(const Complex& c1, const Complex& c2) { // 乘法函数实现
double a = c1.x * c2.x - c1.y * c2.y;
double b = c1.x * c2.y + c1.y * c2.x;
return Complex(a, b);
}
int main() {
Complex c1(2, 3);
Complex c2(4, 5);
cout << "c1: ";
c1.show();
cout << "c2: ";
c2.show();
Complex c3 = add(c1, c2);
cout << "c1 + c2 = ";
c3.show();
Complex c4 = sub(c1, c2);
cout << "c1 - c2 = ";
c4.show();
Complex c5 = mul(c1, c2);
cout << "c1 * c2 = ";
c5.show();
return 0;
}
```
注意,复数类的成员变量 x 和 y 要声明为私有变量,只能通过成员函数访问(例如构造函数、析构函数、复制构造函数和 show 函数)。加法、减法和乘法函数使用了 friend 关键字,使其成为 Complex 类的友元函数,可以直接访问 Complex 类的私有成员变量。程序输出如下:
```
c1: 2 + 3i
c2: 4 + 5i
c1 + c2 = 6 + 8i
c1 - c2 = -2 - 2i
c1 * c2 = -7 + 22i
Complex object destroyed
Complex object destroyed
Complex object destroyed
Complex object destroyed
Complex object destroyed
```
这个程序定义了一个复数类,包括成员变量、构造函数、析构函数、复制构造函数、输出函数和友元函数。在主函数中,通过调用加、减、乘函数实现了复数的加、减、乘运算。在编写复杂的C++代码时,友元函数是一个非常有用的概念,可以与一个类打交道,但不需要暴露类的私有部分。
阅读全文
相关推荐
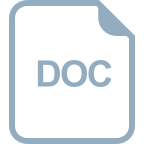
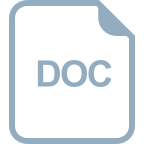













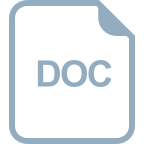
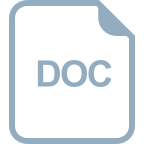
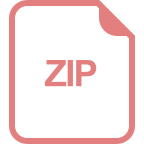
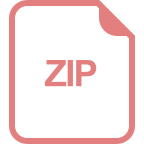