python websockets.serve 中如何设置timeout时间?
时间: 2024-05-14 21:12:54 浏览: 319
可以使用`asyncio.wait_for()`来设置timeout时间,示例代码如下:
```python
import asyncio
import websockets
async def handler(websocket, path):
try:
# 接收数据,设置timeout为5秒
data = await asyncio.wait_for(websocket.recv(), timeout=5)
print(f"Received data: {data}")
except asyncio.TimeoutError:
print("Receive timeout")
async def main():
async with websockets.serve(handler, 'localhost', 8765):
await asyncio.Future() # 防止程序退出
asyncio.run(main())
```
在上面的示例中,`asyncio.wait_for()`函数用于接收数据,如果超过5秒没有接收到数据,就会抛出`asyncio.TimeoutError`异常,从而实现timeout的效果。
相关问题
还是报websockets.exceptions.ConnectionClosedError: sent 1011 (unexpected error) keepalive ping timeout; no close frame received
如果你仍然遇到了相同的错误,那么你可能需要检查一下客户端是否正确地处理了心跳包,以及是否及时回复了服务器发送的心跳包。
另外,你也可以考虑增加一些日志输出,以便更好地排查问题。以下是一个修改后的代码示例,增加了一些日志输出:
```python
import asyncio
import websockets
async def handle_client(websocket, path):
while True:
try:
message = await asyncio.wait_for(websocket.recv(), timeout=50)
print(f"Received message: {message}")
await websocket.send(f"Echoing back your message: {message}")
except asyncio.TimeoutError:
print("Sending keepalive ping...")
await websocket.ping() # 发送心跳包,保持连接状态
except websockets.exceptions.ConnectionClosedError:
print("Connection closed!")
break
async def main():
async with websockets.serve(handle_client, "localhost", 8000):
print("Server started!")
await asyncio.Future() # keep running until Ctrl-C
if __name__ == "__main__":
asyncio.run(main())
```
上述代码中,我们增加了一些针对不同情况的日志输出,以便更好地排查问题。你可以根据实际情况调整日志输出的内容。
这段代码过50秒会报错 错误代码 websockets.exceptions.ConnectionClosedError: sent 1011 (unexpected error) keepalive ping timeout; no close frame received
这个错误是因为 WebSocket 连接没有及时发送心跳包,导致服务器认为连接已经断开而主动关闭了连接。
为了避免这个错误,你可以在服务器端设置一个定时器来定期发送心跳包,以保持连接的活跃状态。以下是一个修改后的代码示例:
```python
import asyncio
import websockets
async def handle_client(websocket, path):
while True:
try:
message = await asyncio.wait_for(websocket.recv(), timeout=50)
print(f"Received message: {message}")
await websocket.send(f"Echoing back your message: {message}")
except asyncio.TimeoutError:
await websocket.ping() # 发送心跳包,保持连接状态
async def main():
async with websockets.serve(handle_client, "localhost", 8000):
print("Server started!")
await asyncio.Future() # keep running until Ctrl-C
if __name__ == "__main__":
asyncio.run(main())
```
上述代码中,我们使用了 `asyncio.wait_for` 函数来设置了一个 50 秒的超时时间,如果在超时时间内没有收到客户端的消息,就会主动发送一个心跳包来保持连接状态。这样可以避免服务器认为连接已经断开而主动关闭连接的情况。
阅读全文
相关推荐
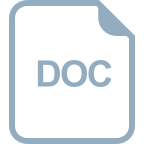
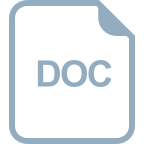
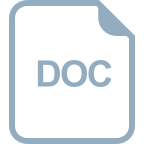
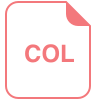
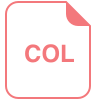
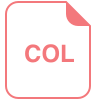
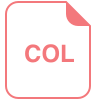
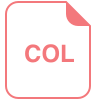
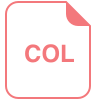
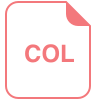
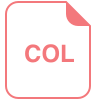
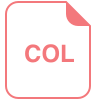
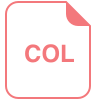
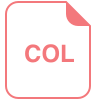
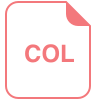
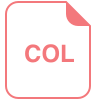