C++矩阵类的加减乘除运算的实现
时间: 2023-08-16 17:05:17 浏览: 101
矩阵类的加减乘除运算可以通过重载运算符来实现。以下是一个矩阵类的定义及其运算符重载实现示例:
```C++
#include <iostream>
#include <vector>
using namespace std;
class Matrix {
public:
Matrix(int rows, int cols) : rows(rows), cols(cols) {
data.resize(rows);
for (int i = 0; i < rows; i++) {
data[i].resize(cols);
}
}
int getRows() const {
return rows;
}
int getCols() const {
return cols;
}
double& operator()(int i, int j) {
return data[i][j];
}
const double& operator()(int i, int j) const {
return data[i][j];
}
Matrix operator+(const Matrix& other) const {
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result(i, j) = (*this)(i, j) + other(i, j);
}
}
return result;
}
Matrix operator-(const Matrix& other) const {
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result(i, j) = (*this)(i, j) - other(i, j);
}
}
return result;
}
Matrix operator*(const Matrix& other) const {
Matrix result(rows, other.cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < other.cols; j++) {
double sum = 0;
for (int k = 0; k < cols; k++) {
sum += (*this)(i, k) * other(k, j);
}
result(i, j) = sum;
}
}
return result;
}
Matrix operator/(const Matrix& other) const {
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result(i, j) = (*this)(i, j) / other(i, j);
}
}
return result;
}
private:
int rows, cols;
vector<vector<double>> data;
};
int main() {
Matrix m1(2, 3);
m1(0, 0) = 1;
m1(0, 1) = 2;
m1(0, 2) = 3;
m1(1, 0) = 4;
m1(1, 1) = 5;
m1(1, 2) = 6;
Matrix m2(3, 2);
m2(0, 0) = 7;
m2(0, 1) = 8;
m2(1, 0) = 9;
m2(1, 1) = 10;
m2(2, 0) = 11;
m2(2, 1) = 12;
Matrix m3 = m1 * m2;
for (int i = 0; i < m3.getRows(); i++) {
for (int j = 0; j < m3.getCols(); j++) {
cout << m3(i, j) << " ";
}
cout << endl;
}
return 0;
}
```
在这个示例中,矩阵类的构造函数接受行数和列数,并动态分配内存来存储矩阵数据。运算符重载实现中,加、减、乘、除分别对应了 `operator+`、`operator-`、`operator*`、`operator/`。`operator()` 用于访问矩阵元素。在 main 函数中,首先创建了两个矩阵 m1 和 m2,然后计算它们的乘积,并输出结果。
相关推荐
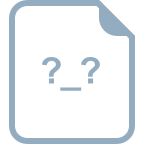
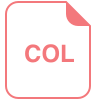













