实数矩阵类的加减乘除运算的实现C++
时间: 2023-08-16 21:04:30 浏览: 121
以下是一个简单的实数矩阵类的加减乘除运算的实现示例:
```cpp
#include <iostream>
#include <vector>
using namespace std;
class Matrix {
public:
Matrix(int rows, int cols) {
this->rows = rows;
this->cols = cols;
data.resize(rows, vector<double>(cols));
}
Matrix operator+(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw runtime_error("Matrix dimensions must match.");
}
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
Matrix operator-(const Matrix& other) const {
if (rows != other.rows || cols != other.cols) {
throw runtime_error("Matrix dimensions must match.");
}
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] - other.data[i][j];
}
}
return result;
}
Matrix operator*(const Matrix& other) const {
if (cols != other.rows) {
throw runtime_error("Matrix dimensions must match.");
}
Matrix result(rows, other.cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < other.cols; j++) {
double sum = 0;
for (int k = 0; k < cols; k++) {
sum += data[i][k] * other.data[k][j];
}
result.data[i][j] = sum;
}
}
return result;
}
Matrix operator/(double scalar) const {
Matrix result(rows, cols);
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
result.data[i][j] = data[i][j] / scalar;
}
}
return result;
}
double& operator()(int row, int col) {
return data[row][col];
}
const double& operator()(int row, int col) const {
return data[row][col];
}
void print() const {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cout << data[i][j] << " ";
}
cout << endl;
}
}
private:
int rows, cols;
vector<vector<double>> data;
};
```
这个类定义了一个矩阵对象,可以通过构造函数指定矩阵的行数和列数。它还重载了加、减、乘、除等运算符,以便可以像操作整数或浮点数一样操作矩阵。其中,加、减、乘运算都需要检查矩阵维度是否匹配,如果不匹配则抛出异常。此外,它还重载了括号运算符,以便可以像访问数组元素一样访问矩阵元素。最后,它还定义了一个打印矩阵的方法。
以下是一个示例程序,演示如何使用这个类进行矩阵运算:
```cpp
int main() {
Matrix A(2, 2);
A(0, 0) = 1;
A(0, 1) = 2;
A(1, 0) = 3;
A(1, 1) = 4;
Matrix B(2, 2);
B(0, 0) = 5;
B(0, 1) = 6;
B(1, 0) = 7;
B(1, 1) = 8;
Matrix C = A + B;
cout << "A + B = " << endl;
C.print();
Matrix D = A - B;
cout << "A - B = " << endl;
D.print();
Matrix E = A * B;
cout << "A * B = " << endl;
E.print();
Matrix F = A / 2;
cout << "A / 2 = " << endl;
F.print();
return 0;
}
```
输出结果如下:
```
A + B =
6 8
10 12
A - B =
-4 -4
-4 -4
A * B =
19 22
43 50
A / 2 =
0.5 1
1.5 2
```
以上就是一个简单的实数矩阵类的加减乘除运算的实现示例。当然,这只是一个基础的实现,实际应用中可能需要考虑更多的情况,如矩阵的转置、求逆、行列式等操作,以及优化矩阵乘法的运算效率等。
阅读全文
相关推荐
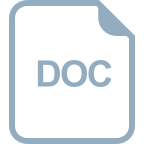
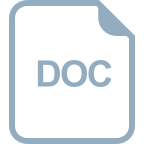
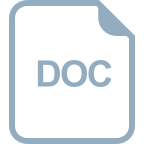
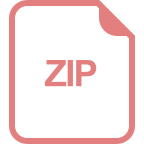
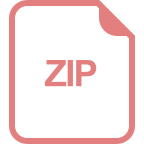
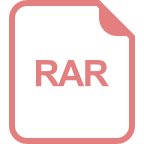
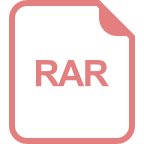
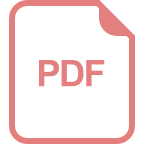
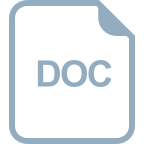
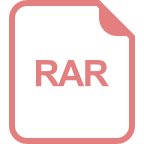
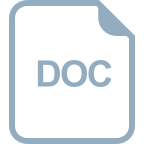
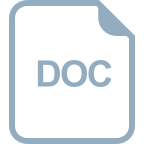
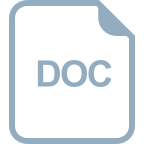
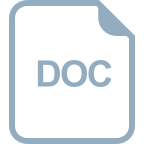
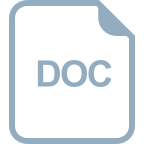
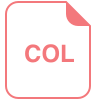
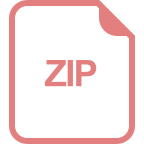
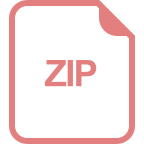