请利用insert、update、delete触发器,实现仓库管理系统的库存实时计算,包含产品表、入库表、出库表、库存表,完成设计思路和SQL程序实现
时间: 2023-10-20 21:19:57 浏览: 68
设计思路:
1. 创建四个表,分别为产品表(product)、入库表(in_storage)、出库表(out_storage)和库存表(inventory)。
2. 产品表包含产品编号、产品名称、产品规格等信息。
3. 入库表包含入库单号、产品编号、入库时间、入库数量等信息。
4. 出库表包含出库单号、产品编号、出库时间、出库数量等信息。
5. 库存表包含产品编号、库存数量等信息。
6. 创建三个触发器,分别为入库触发器、出库触发器和更新库存触发器。
7. 入库触发器在插入入库表数据时,根据产品编号更新库存表中对应产品的库存数量。
8. 出库触发器在插入出库表数据时,根据产品编号更新库存表中对应产品的库存数量。
9. 更新库存触发器在更新入库表或出库表时,重新计算库存表中对应产品的库存数量。
SQL程序实现:
创建产品表
```sql
CREATE TABLE product (
product_id INT PRIMARY KEY,
product_name VARCHAR(50) NOT NULL,
product_specification VARCHAR(50) NOT NULL
);
```
创建入库表
```sql
CREATE TABLE in_storage (
in_storage_id INT PRIMARY KEY,
product_id INT NOT NULL,
in_storage_time DATETIME NOT NULL,
in_storage_quantity INT NOT NULL,
FOREIGN KEY (product_id) REFERENCES product(product_id)
);
```
创建出库表
```sql
CREATE TABLE out_storage (
out_storage_id INT PRIMARY KEY,
product_id INT NOT NULL,
out_storage_time DATETIME NOT NULL,
out_storage_quantity INT NOT NULL,
FOREIGN KEY (product_id) REFERENCES product(product_id)
);
```
创建库存表
```sql
CREATE TABLE inventory (
product_id INT PRIMARY KEY,
inventory_quantity INT NOT NULL,
FOREIGN KEY (product_id) REFERENCES product(product_id)
);
```
创建入库触发器
```sql
CREATE TRIGGER update_inventory_on_insert_in_storage
AFTER INSERT ON in_storage
FOR EACH ROW
BEGIN
UPDATE inventory
SET inventory_quantity = inventory_quantity + NEW.in_storage_quantity
WHERE product_id = NEW.product_id;
END;
```
创建出库触发器
```sql
CREATE TRIGGER update_inventory_on_insert_out_storage
AFTER INSERT ON out_storage
FOR EACH ROW
BEGIN
UPDATE inventory
SET inventory_quantity = inventory_quantity - NEW.out_storage_quantity
WHERE product_id = NEW.product_id;
END;
```
创建更新库存触发器
```sql
CREATE TRIGGER update_inventory_on_update
AFTER UPDATE ON in_storage, out_storage
FOR EACH ROW
BEGIN
DECLARE total_in_storage INT;
DECLARE total_out_storage INT;
SELECT SUM(in_storage_quantity) INTO total_in_storage FROM in_storage WHERE product_id = NEW.product_id;
SELECT SUM(out_storage_quantity) INTO total_out_storage FROM out_storage WHERE product_id = NEW.product_id;
UPDATE inventory
SET inventory_quantity = total_in_storage - total_out_storage
WHERE product_id = NEW.product_id;
END;
```
相关推荐
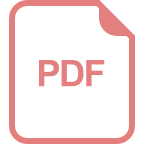
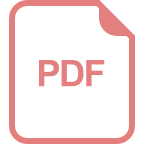
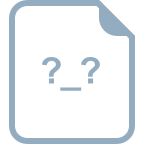
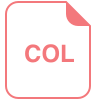
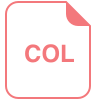
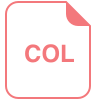











