使用Servlet、DAO、和VO完成对学生信息的查询。 注:在MySql数据库DSSchool的t_student表中保存有学生姓名、学号等信息。
时间: 2024-05-15 17:12:47 浏览: 205
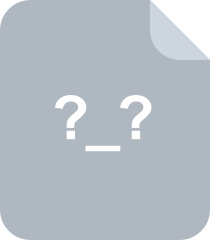
基于Servlet的学生表查询
首先,我们需要创建一个VO类来表示学生信息:
```java
public class Student {
private String name;
private String id;
// 构造方法和getter/setter方法省略
}
```
然后,我们需要创建一个DAO类来访问数据库:
```java
public class StudentDao {
private Connection conn;
public StudentDao(Connection conn) {
this.conn = conn;
}
public List<Student> findAll() throws SQLException {
List<Student> students = new ArrayList<>();
PreparedStatement stmt = conn.prepareStatement("SELECT * FROM t_student");
ResultSet rs = stmt.executeQuery();
while (rs.next()) {
Student student = new Student();
student.setName(rs.getString("name"));
student.setId(rs.getString("id"));
students.add(student);
}
rs.close();
stmt.close();
return students;
}
}
```
最后,我们需要创建一个Servlet来处理查询请求:
```java
public class StudentServlet extends HttpServlet {
private StudentDao studentDao;
public void init() throws ServletException {
try {
Class.forName("com.mysql.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost/DSSchool", "username", "password");
studentDao = new StudentDao(conn);
} catch (ClassNotFoundException | SQLException e) {
throw new ServletException(e);
}
}
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
try {
List<Student> students = studentDao.findAll();
request.setAttribute("students", students);
request.getRequestDispatcher("/student.jsp").forward(request, response);
} catch (SQLException e) {
throw new ServletException(e);
}
}
}
```
其中,我们将查询结果保存在request属性中,然后将请求转发到一个JSP页面来展示结果:
```jsp
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>学生信息查询</title>
</head>
<body>
<h1>学生信息查询结果</h1>
<table>
<thead>
<tr>
<th>姓名</th>
<th>学号</th>
</tr>
</thead>
<tbody>
<c:forEach var="student" items="${students}">
<tr>
<td>${student.name}</td>
<td>${student.id}</td>
</tr>
</c:forEach>
</tbody>
</table>
</body>
</html>
```
这样,当我们访问这个Servlet时,就会查询数据库并展示查询结果。
阅读全文
相关推荐
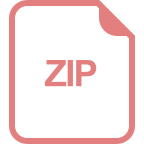
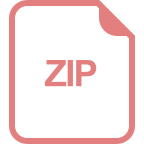
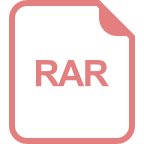
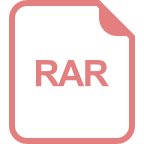
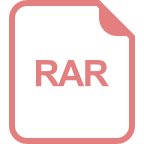
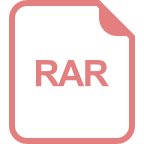
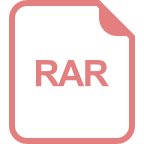
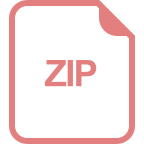
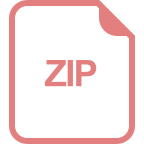
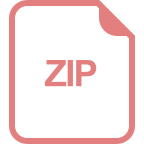
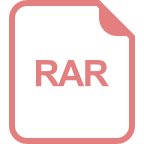
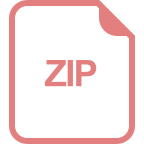
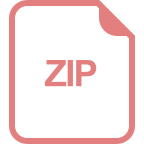
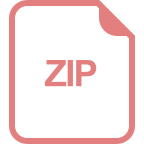
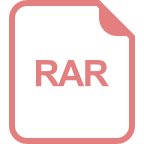
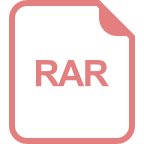