N = 5student = [None] * Ndef input_stu(stu): for i in range(N): student[i] = []def output_stu(stu): print(student)if __name__ == '__main__': input_stu(student) output_stu(student)
时间: 2023-07-15 14:13:47 浏览: 130
这段代码定义了一个长度为5的列表`student`,然后定义了两个函数`input_stu`和`output_stu`。
`input_stu`函数将输入的`stu`添加到`student`列表中。具体地,它使用`for`循环迭代`range(N)`,在每次迭代中将一个空列表添加到`student`中。这样,`student`列表中就有了5个空列表。
`output_stu`函数打印出整个`student`列表,包括所有5个空列表。
最后,通过`if __name__ == '__main__':`语句将`input_stu`和`output_stu`函数应用于`student`列表。
相关问题
清单action android:name="android.nfc.action.NDEF_DISCOVERED怎么用
`android.nfc.action.NDEF_DISCOVERED` 是一种 NFC 技术规范,它指定了当系统检测到一个 NDEF 类型的标签或卡片被放置在 NFC 读写器附近时,应该启动哪个应用程序来处理这个标签或卡片。
要使用 `android.nfc.action.NDEF_DISCOVERED`,你需要在你的应用程序的清单文件中指定相应的 intent-filter。例如:
```
<activity android:name=".MyNFCActivity">
<intent-filter>
<action android:name="android.nfc.action.NDEF_DISCOVERED" />
<category android:name="android.intent.category.DEFAULT" />
<data android:mimeType="text/plain" />
</intent-filter>
</activity>
```
上面的代码片段中,`MyNFCActivity` 是你的应用程序中处理 NFC 数据的活动(Activity),`android.nfc.action.NDEF_DISCOVERED` 是指定的 intent-action,`android.intent.category.DEFAULT` 是指定的 intent-category,`text/plain` 是指定的数据类型。这个 intent-filter 的含义是,当系统检测到一个 NDEF 类型的标签或卡片被放置在 NFC 读写器附近时,并且这个标签或卡片中包含了文本数据,系统就会启动 `MyNFCActivity` 来处理这个数据。
在你的活动(Activity)中,你可以通过 `getIntent()` 方法获取传递给这个活动的 intent,然后通过 `getParcelableExtra(NfcAdapter.EXTRA_TAG)` 方法获取标签或卡片的实例,然后读取标签或卡片中的数据。
需要注意的是,`android.nfc.action.NDEF_DISCOVERED` 只是一种 NFC 数据处理方式,你还可以使用其他的 intent-action 和 intent-filter 来处理其他类型的 NFC 数据。同时,不同的 NFC 标签或卡片可能有不同的数据格式和读取方式,因此在处理 NFC 数据时需要先了解相关的技术规范和数据格式。
import React, { Component } from 'react'; import { Text, View } from 'react-native'; import NfcManager, { NfcTech, Ndef } from 'react-native-nfc-manager'; export default class App extends Component { state = { message: '', }; componentDidMount() { NfcManager.start(); NfcManager.setEventListener(NfcManager.EVENT_NDEF_DISCOVERED, this.onNfcReceived); } componentWillUnmount() { NfcManager.setEventListener(NfcManager.EVENT_NDEF_DISCOVERED, null); NfcManager.stop(); } onNfcReceived = async (tag) => { const ndef = await NfcManager.ndefHandler.parseUri(tag.ndefMessage[0].payload); this.setState({ message: ndef }); }; sendNfcMessage = async () => { try { await NfcManager.requestTechnology(NfcTech.Ndef, { alertMessage: 'Ready to send NFC message', }); const bytes = Ndef.encodeMessage([Ndef.textRecord('Hello, world!')]); await NfcManager.ndefHandler.writeNdefMessage(bytes); NfcManager.cancelTechnologyRequest(); } catch (error) { console.warn('Error sending NFC message', error); } }; render() { return ( <View> <Text>{this.state.message}</Text> <Button title="Send NFC Message" onPress={this.sendNfcMessage} /> </View> ); } }优化上面的代码,编译没有通过
根据您提供的代码,我看到您没有导入 `Button` 组件,这可能会导致编译错误。您可以通过以下方式导入 `Button` 组件:
```javascript
import { Text, View, Button } from 'react-native';
```
另外,您在写 `setState` 时,需要将 `ndef` 对象中的 `uri` 属性作为参数传递给 `setState`,而不是直接将 `ndef` 对象作为参数传递。因此,您需要将 `onNfcReceived` 函数中的代码修改为以下内容:
```javascript
onNfcReceived = async (tag) => {
const ndef = await NfcManager.ndefHandler.parseUri(tag.ndefMessage[0].payload);
this.setState({ message: ndef.uri });
};
```
最后,为了避免可能出现的内存泄漏,建议您在组件卸载时手动移除 `NfcManager` 的事件监听器。因此,您需要将 `componentWillUnmount` 函数中的代码修改为以下内容:
```javascript
componentWillUnmount() {
NfcManager.setEventListener(NfcManager.EVENT_NDEF_DISCOVERED, null);
NfcManager.unregisterTagEvent();
NfcManager.stop();
}
```
修改后的代码如下:
```javascript
import React, { Component } from 'react';
import { Text, View, Button } from 'react-native';
import NfcManager, { NfcTech, Ndef } from 'react-native-nfc-manager';
export default class App extends Component {
state = {
message: '',
};
componentDidMount() {
NfcManager.start();
NfcManager.setEventListener(NfcManager.EVENT_NDEF_DISCOVERED, this.onNfcReceived);
}
componentWillUnmount() {
NfcManager.setEventListener(NfcManager.EVENT_NDEF_DISCOVERED, null);
NfcManager.unregisterTagEvent();
NfcManager.stop();
}
onNfcReceived = async (tag) => {
const ndef = await NfcManager.ndefHandler.parseUri(tag.ndefMessage[0].payload);
this.setState({ message: ndef.uri });
};
sendNfcMessage = async () => {
try {
await NfcManager.requestTechnology(NfcTech.Ndef, {
alertMessage: 'Ready to send NFC message',
});
const bytes = Ndef.encodeMessage([Ndef.textRecord('Hello, world!')]);
await NfcManager.ndefHandler.writeNdefMessage(bytes);
NfcManager.cancelTechnologyRequest();
} catch (error) {
console.warn('Error sending NFC message', error);
}
};
render() {
return (
<View>
<Text>{this.state.message}</Text>
<Button title="Send NFC Message" onPress={this.sendNfcMessage} />
</View>
);
}
}
```
希望这些修改能够帮助您解决问题。
阅读全文
相关推荐
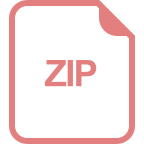
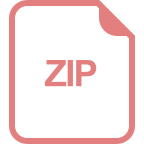
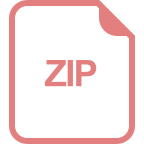
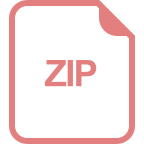
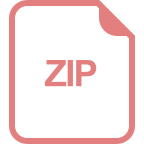
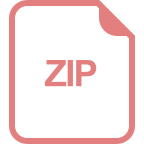
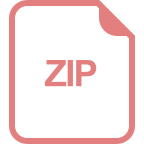
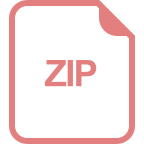
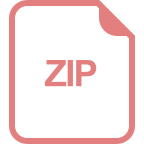
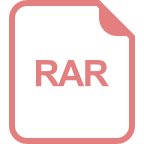
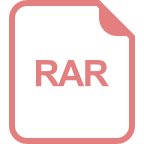
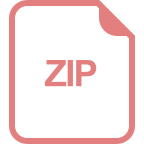
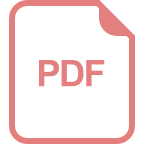
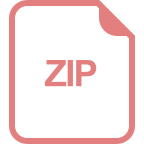
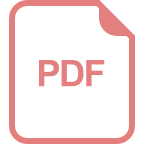
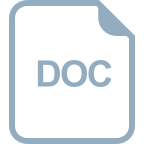
