vue中template获取数组长度
时间: 2023-08-30 14:06:27 浏览: 246
在 Vue 的模板中,获取数组的长度可以使用 JavaScript 的 `Array.length` 属性。例如:
```html
<template>
<div>
<p>数组长度为:{{ items.length }}</p>
<ul>
<li v-for="(item, index) in items" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: ['apple', 'banana', 'orange']
}
}
}
</script>
```
在上面的例子中,`{{ items.length }}` 表达式可以获取 `items` 数组的长度。然后,我们使用 `v-for` 指令遍历 `items` 数组中的每个元素,并在模板中显示它们。
相关问题
Vue 判断后端的数组长度
你可以使用Vue的计算属性来判断后端数组的长度。首先,在Vue组件中定义一个计算属性,例如"arrayLength",然后在该计算属性中返回后端数组的长度。这样,每当后端数组发生变化时,计算属性会自动更新。下面是一个示例:
```javascript
<template>
<div>
<p v-if="arrayLength === 0">后端数组为空</p>
<p v-else>后端数组长度为 {{ arrayLength }}</p>
</div>
</template>
<script>
export default {
data() {
return {
backendArray: [], // 后端数组
};
},
computed: {
arrayLength() {
return this.backendArray.length;
},
},
};
</script>
```
在上面的示例中,我们在模板中使用了Vue的条件渲染指令(v-if和v-else)来根据后端数组的长度显示不同的内容。如果后端数组的长度为0,则显示"后端数组为空",否则显示"后端数组长度为 X",其中X是后端数组的实际长度。
请注意,这只是一个示例,你需要根据你的实际情况来修改代码。同时,确保你已经正确获取后端数组的数据,并将其存储在组件的data属性中。
vue中正则验证数组长度
你可以使用 Vue 中的自定义指令来实现对数组长度的正则验证。下面是一个简单的例子:
```javascript
Vue.directive('array-length', {
bind: function (el, binding) {
el.addEventListener('input', function () {
const regex = binding.value.regex;
const maxLength = binding.value.maxLength;
const value = el.value;
if (Array.isArray(value) && value.length > maxLength) {
el.setCustomValidity(`数组长度不能超过 ${maxLength}`);
return;
}
if (!regex.test(value)) {
el.setCustomValidity('格式不正确');
return;
}
el.setCustomValidity('');
});
}
})
```
然后在你的模板中,你可以使用这个指令来验证你的数组:
```html
<template>
<input type="text" v-model="myArray" v-array-length="{ regex: /your-regex-here/, maxLength: 5 }">
</template>
```
这个例子中,我们定义了一个 `v-array-length` 指令,它接受一个包含正则表达式和最大长度的对象作为参数。当输入框的值改变时,我们使用这些参数来验证数组的长度和格式。如果验证失败,我们使用 `setCustomValidity` 方法来设置输入框的自定义验证错误信息。
阅读全文
相关推荐
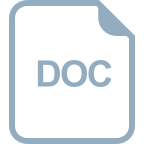
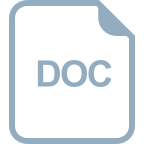
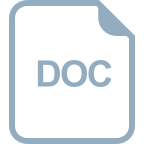













