read_packet从fifo中读取给个示例
时间: 2024-03-09 13:48:37 浏览: 32
下面是一个简单的示例,展示了如何通过 FIFO 从 `read_packet` 函数中读取数据:
```c
#include <stdio.h>
#include <stdlib.h>
#include <libavformat/avformat.h>
#define FIFO_SIZE 1024
typedef struct {
uint8_t *data;
int size;
int pos;
} Buffer;
int read_packet(void *opaque, uint8_t *buf, int buf_size) {
AVFifoBuffer *fifo = (AVFifoBuffer *)opaque;
int bytes_left = av_fifo_size(fifo);
int bytes_to_copy = buf_size < bytes_left ? buf_size : bytes_left;
if (bytes_to_copy == 0) {
return AVERROR_EOF;
}
av_fifo_generic_read(fifo, buf, bytes_to_copy, NULL);
return bytes_to_copy;
}
int main(int argc, char *argv[]) {
av_register_all();
AVFifoBuffer *fifo = av_fifo_alloc(FIFO_SIZE);
if (!fifo) {
fprintf(stderr, "failed to allocate FIFO\n");
return 1;
}
// 从 FIFO 中写入数据
char *data = "Hello, world!";
int size = strlen(data);
av_fifo_generic_write(fifo, data, size, NULL);
AVIOContext *avio_ctx = NULL;
avio_ctx = avio_alloc_context(
(uint8_t *)malloc(BUFFER_SIZE),
BUFFER_SIZE,
0,
fifo,
&read_packet,
NULL,
NULL
);
AVFormatContext *avfmt_ctx = NULL;
int ret = avformat_open_input(&avfmt_ctx, "", NULL, NULL);
if (ret < 0) {
fprintf(stderr, "failed to open input: %s\n", av_err2str(ret));
return 1;
}
avfmt_ctx->pb = avio_ctx;
ret = avformat_find_stream_info(avfmt_ctx, NULL);
if (ret < 0) {
fprintf(stderr, "failed to find stream info: %s\n", av_err2str(ret));
return 1;
}
av_dump_format(avfmt_ctx, 0, "", 0);
avformat_close_input(&avfmt_ctx);
avio_context_free(&avio_ctx);
av_fifo_free(fifo);
return 0;
}
```
在这个例子中,我们创建了一个大小为 `FIFO_SIZE` 的 FIFO,并向其中写入了一些数据。然后我们将 FIFO 传递给 `read_packet` 函数,使得它可以从 FIFO 中读取数据。在 `read_packet` 函数中,我们使用 `av_fifo_generic_read` 函数从 FIFO 中读取数据。你可以将 `AVFifoBuffer` 结构体换成你自己的 FIFO 实现,然后在 `read_packet` 函数中读取数据即可。
相关推荐
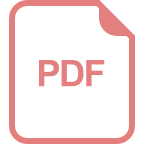
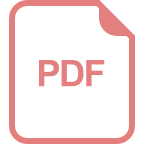














