stm32麦克纳姆轮小车pid控制程序
时间: 2023-06-12 10:07:49 浏览: 128
下面是一个基于STM32的麦克纳姆轮小车PID控制程序的示例,供参考:
首先,需要定义一些常量和变量:
```c
#define PI 3.14159265358979323846f
#define WHEEL_DIAMETER 0.05f // 轮子直径
#define WHEEL_DISTANCE 0.2f // 轮子间距
float pid_kp = 1.0f; // PID控制器的比例系数
float pid_ki = 0.0f; // PID控制器的积分系数
float pid_kd = 0.0f; // PID控制器的微分系数
float target_velocity_x = 0.0f; // 目标x轴速度
float target_velocity_y = 0.0f; // 目标y轴速度
float target_angular_velocity = 0.0f; // 目标角速度
float current_velocity_x = 0.0f; // 当前x轴速度
float current_velocity_y = 0.0f; // 当前y轴速度
float current_angular_velocity = 0.0f; // 当前角速度
float velocity_error_x = 0.0f; // x轴速度误差
float velocity_error_y = 0.0f; // y轴速度误差
float angular_velocity_error = 0.0f; // 角速度误差
float velocity_error_integral_x = 0.0f; // x轴速度误差积分
float velocity_error_integral_y = 0.0f; // y轴速度误差积分
float angular_velocity_error_integral = 0.0f; // 角速度误差积分
float velocity_error_previous_x = 0.0f; // 用于微分的上一次速度误差
float velocity_error_previous_y = 0.0f; // 用于微分的上一次速度误差
float angular_velocity_error_previous = 0.0f; // 用于微分的上一次角速度误差
float pwm_motor1 = 0.0f; // 电机1的PWM输出
float pwm_motor2 = 0.0f; // 电机2的PWM输出
float pwm_motor3 = 0.0f; // 电机3的PWM输出
float pwm_motor4 = 0.0f; // 电机4的PWM输出
float wheel_speed1 = 0.0f; // 电机1输出的轮速
float wheel_speed2 = 0.0f; // 电机2输出的轮速
float wheel_speed3 = 0.0f; // 电机3输出的轮速
float wheel_speed4 = 0.0f; // 电机4输出的轮速
uint32_t last_time = 0; // 上一次计算PID的时间
```
然后,需要编写一个PID计算函数,用来根据误差计算出PWM输出:
```c
void pid_compute(float *pwm1, float *pwm2, float *pwm3, float *pwm4, uint32_t current_time)
{
float delta_time = (current_time - last_time) / 1000.0f;
velocity_error_x = target_velocity_x - current_velocity_x;
velocity_error_y = target_velocity_y - current_velocity_y;
angular_velocity_error = target_angular_velocity - current_angular_velocity;
velocity_error_integral_x += velocity_error_x * delta_time;
velocity_error_integral_y += velocity_error_y * delta_time;
angular_velocity_error_integral += angular_velocity_error * delta_time;
float velocity_error_derivative_x = (velocity_error_x - velocity_error_previous_x) / delta_time;
float velocity_error_derivative_y = (velocity_error_y - velocity_error_previous_y) / delta_time;
float angular_velocity_error_derivative = (angular_velocity_error - angular_velocity_error_previous) / delta_time;
*pwm1 = pid_kp * velocity_error_x + pid_ki * velocity_error_integral_x + pid_kd * velocity_error_derivative_x;
*pwm2 = pid_kp * velocity_error_y + pid_ki * velocity_error_integral_y + pid_kd * velocity_error_derivative_y;
*pwm3 = pid_kp * angular_velocity_error + pid_ki * angular_velocity_error_integral + pid_kd * angular_velocity_error_derivative;
*pwm4 = 0.0f; // 第四个电机不使用
velocity_error_previous_x = velocity_error_x;
velocity_error_previous_y = velocity_error_y;
angular_velocity_error_previous = angular_velocity_error;
last_time = current_time;
}
```
最后,在主循环中,读取传感器数据,计算当前速度和角速度,然后调用PID计算函数计算PWM输出:
```c
while (1)
{
// 读取传感器数据
float imu_heading = read_imu_heading();
float encoder1 = read_encoder1();
float encoder2 = read_encoder2();
float encoder3 = read_encoder3();
float encoder4 = read_encoder4();
// 计算当前速度和角速度
float wheel_speeds[4] = { 0 };
wheel_speeds[0] = (encoder1 / 360.0f) * WHEEL_DIAMETER * PI;
wheel_speeds[1] = (encoder2 / 360.0f) * WHEEL_DIAMETER * PI;
wheel_speeds[2] = (encoder3 / 360.0f) * WHEEL_DIAMETER * PI;
wheel_speeds[3] = (encoder4 / 360.0f) * WHEEL_DIAMETER * PI;
current_velocity_x = (-wheel_speeds[0] + wheel_speeds[1] + wheel_speeds[2] - wheel_speeds[3]) / 4.0f;
current_velocity_y = (wheel_speeds[0] + wheel_speeds[1] - wheel_speeds[2] - wheel_speeds[3]) / 4.0f;
current_angular_velocity = (wheel_speeds[0] + wheel_speeds[1] + wheel_speeds[2] + wheel_speeds[3]) / (4.0f * WHEEL_DISTANCE);
// 调用PID计算函数计算PWM输出
uint32_t current_time = get_current_time();
pid_compute(&pwm_motor1, &pwm_motor2, &pwm_motor3, &pwm_motor4, current_time);
// 输出PWM信号到电机
set_motor_pwm(MOTOR1, pwm_motor1);
set_motor_pwm(MOTOR2, pwm_motor2);
set_motor_pwm(MOTOR3, pwm_motor3);
set_motor_pwm(MOTOR4, pwm_motor4);
}
```
需要注意的是,这只是一个简单的示例,实际应用中需要根据具体情况进行调整和改进。
相关推荐
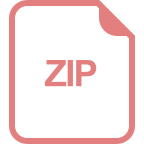
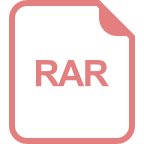
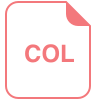
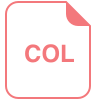
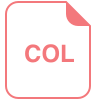
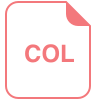
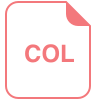









